JavaScript is a versatile and powerful programming language that is primarily used for creating interactive and dynamic web pages. It was created by Brendan Eich in 1995 while he was working at Netscape Communications Corporation. Initially, JavaScript was developed as a way to add interactivity to static HTML pages, but over the years, it has evolved into a full-fledged programming language that can be used for both front-end and back-end development.
JavaScript, often abbreviated as JS, has become one of the most popular programming languages in the world. It was initially developed by Brendan Eich at Netscape Communications Corporation in 1995. Since then, it has evolved significantly and is now supported by all major web browsers, making it an essential tool for web developers.
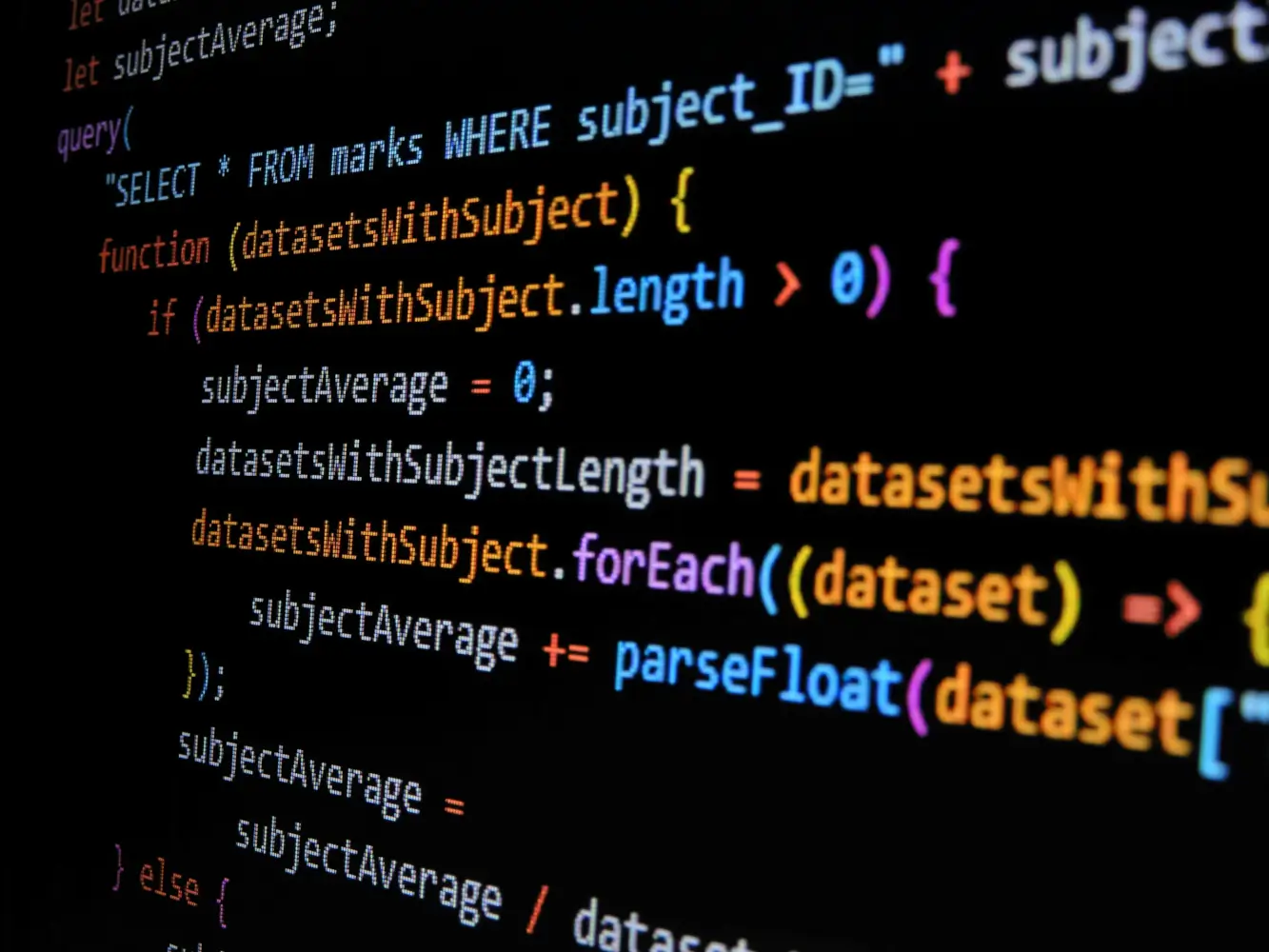
Why Java Script is essential to learn
One of the key features of JavaScript is its ability to run directly in the browser, without the need for any additional software or plugins. This means that users can interact with JavaScript-powered websites without having to install anything on their devices. This is in stark contrast to other programming languages, such as Java or C++, which require the installation of a runtime environment.
JavaScript is a versatile language that can be used for a wide range of tasks. It can be used to validate form inputs, create interactive maps, build games, and even develop complex web applications. Its flexibility and ease of use make it an ideal choice for both beginner and experienced developers.
The Document Object Model (DOM)
Integration with HTML and CSS
One of the main reasons for JavaScript’s popularity is its integration with HTML and CSS. HTML provides the structure of a web page, CSS takes care of the presentation, and JavaScript handles the behavior. This combination allows developers to create rich and interactive user experiences.
client-side form validation
JavaScript is also widely used for client-side form validation. With JavaScript, you can write code that checks whether the user has entered valid data in a form before submitting it to the server. This can help improve the user experience by providing instant feedback to the user and reducing the number of server-side validation requests.
Supported by all modern web browsers
Over the years, JavaScript has become an essential part of web development. It is supported by all modern web browsers and has a large and active community of developers. JavaScript frameworks and libraries, such as React, Angular, and jQuery, have also emerged, making it easier to build complex web applications.
Event-driven nature
One of the key features of JavaScript is its event-driven nature, which means that it can respond to user actions such as button clicks, form submissions, and mouse movements. This allows developers to create interactive elements like dropdown menus, sliders, and carousels that enhance the user experience.
Events are actions or occurrences that happen in the browser, such as a button click or a page load. JavaScript allows developers to write code that responds to these events and perform certain actions accordingly. For example, you can write JavaScript code that displays an alert message when a button is clicked or validates user input in a form.
Libraries and frameworks
Furthermore, JavaScript has an extensive ecosystem of libraries and frameworks that simplify the development process. These tools provide pre-built functionality and components, allowing developers to focus on solving specific problems rather than reinventing the wheel.
With the rise of technologies like Node.js, React, and Angular, JavaScript has become not only a language for the web but also a popular choice for server-side and mobile app development. Its versatility and wide range of frameworks and libraries make it a powerful tool for building modern applications. More on Libraries later.
Core Concepts of JavaScript
Before we dive into the syntax and basic constructs of JavaScript, let’s understand some core concepts that form the foundation of the language:
- Variables and Data Types: JavaScript allows you to store and manipulate data using variables. It supports various data types such as numbers, strings, booleans, arrays, objects, and more.
- Control Structures: JavaScript provides control structures like loops and conditionals to control the flow of your program. Loops, such as the for loop and while loop, allow you to repeat a block of code multiple times based on a condition. Conditionals, such as if statements and switch statements, enable you to execute different blocks of code based on different conditions.
- Functions and Event Handling: Functions are reusable blocks of code that perform a specific task. JavaScript allows you to define and invoke functions. You can pass arguments to functions, which are variables that hold values that are passed to the function when it is invoked. Functions can also return values, which can be used in other parts of your program. Event handling enables you to respond to user actions, such as clicking a button or submitting a form. You can attach event handlers to HTML elements, and when the specified event occurs, the associated JavaScript code is executed.
Syntax and Basic Constructs
Now that we have a basic understanding of JavaScript’s core concepts, let’s explore its syntax and some basic constructs:
- Variables: In JavaScript, you can declare variables using the
var
,let
, orconst
keyword. Variables declared withvar
have function scope, while variables declared withlet
andconst
have block scope. This means that variables declared withvar
are accessible throughout the entire function in which they are declared, while variables declared withlet
andconst
are only accessible within the block of code in which they are defined. Additionally, variables declared withconst
cannot be reassigned once they have been assigned a value. - Data Types: JavaScript has several data types, including numbers, strings, booleans, arrays, objects, and more. Numbers can be both integers and floating-point numbers, and you can perform mathematical operations on them. Strings are sequences of characters and can be enclosed in single or double quotes. Booleans represent either true or false values. Arrays are ordered lists of values, and objects are collections of key-value pairs. You can perform operations and manipulate data using these data types. JavaScript also has special data types like null and undefined.
- Control Structures: JavaScript provides various control structures to control the flow of your program based on certain conditions.
If
statements allow you to execute a block of code if a certain condition is true. You can also useelse if
andelse
statements to specify alternative conditions.For
andwhile
loops allow you to repeat a block of code multiple times. Theswitch
statement allows you to perform different actions based on different conditions. Control structures are essential for creating dynamic and interactive programs.
Event Handling
Event handling is another important aspect of JavaScript. It allows you to respond to user actions such as clicking a button or submitting a form. By attaching event listeners to HTML elements, you can execute specific code when the event occurs. Event handling is crucial for creating interactive web pages and enhancing user experience.
To handle events, you can use the `addEventListener` method, which takes two parameters: the event type and the function to be executed when the event occurs. For example, if you want to execute a function when a button is clicked, you can add an event listener to the button element with the event type of “click” and the function to be executed.
In addition to the `click` event, there are many other types of events you can handle, such as `mouseover`, `keydown`, `submit`, and `load`. Each event type corresponds to a specific user action or an action triggered by the browser.
Event handling also allows you to access information about the event itself. For example, when handling a click event, you can access the target element that was clicked using the `event.target` property. This allows you to perform different actions based on which element was clicked.
const button = document.querySelector('button');
button.addEventListener('click', (event) => {
console.log(event.target); // outputs the button element that was clicked
});
In addition to attaching event listeners to individual elements, you can also handle events at the document level. This is useful when you want to handle events for multiple elements or dynamically created elements. By attaching event listeners to the document, you can capture events that occur within the entire document.
document.addEventListener('click', (event) => {
console.log(event.target); // outputs the element that was clicked, regardless of its type
});
Overall, functions and event handling are essential concepts in JavaScript. Functions allow you to organize your code into reusable blocks, while event handling enables you to create interactive web pages that respond to user actions. Mastering these concepts will greatly enhance your ability to create dynamic and engaging web applications.
DOM Manipulation and Web APIs
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a web page and allows JavaScript to interact with the elements on the page. Let’s explore some basic DOM manipulation techniques:
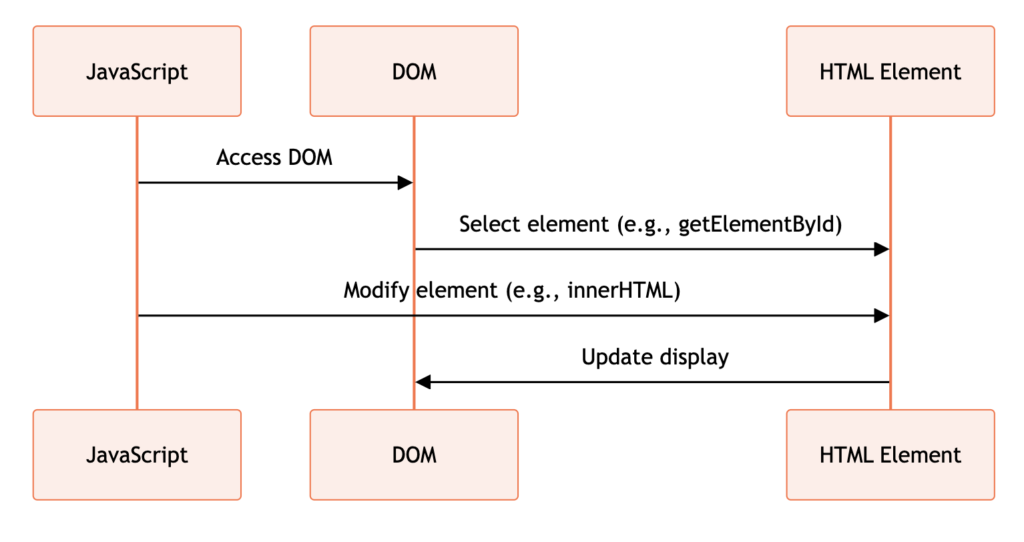
- What is the DOM? The DOM is a tree-like structure that represents the elements of an HTML document. It provides methods and properties to access and manipulate these elements.
- Basic DOM Manipulation Techniques: JavaScript provides methods like
getElementById
,getElementsByClassName
, andquerySelector
to select and manipulate HTML elements. You can change their content, style, attributes, and more. - Traversing the DOM: In addition to selecting elements, you can also traverse the DOM using methods like
parentNode
,childNodes
, andnextSibling
. These methods allow you to access and manipulate elements based on their relationship with other elements in the DOM tree. - Modifying the DOM: Once you have selected an element, you can modify its content using properties like
innerHTML
andtextContent
. You can also add or remove elements using methods likeappendChild
,removeChild
, andcreateElement
.
Web APIs (Application Programming Interfaces) are interfaces provided by web browsers that allow JavaScript to interact with various web features and services. Let’s have a brief introduction to web APIs:
- Introduction to Web APIs: Web APIs provide functionality beyond the basic DOM manipulation. They allow you to work with features like fetching data from external sources (AJAX), manipulating images, playing audio and video, and more.
- Popular Web APIs: Some popular web APIs include the Fetch API, which allows you to make HTTP requests and handle responses, the Geolocation API, which allows you to retrieve the user’s location, and the Web Storage API, which allows you to store data locally in the browser.
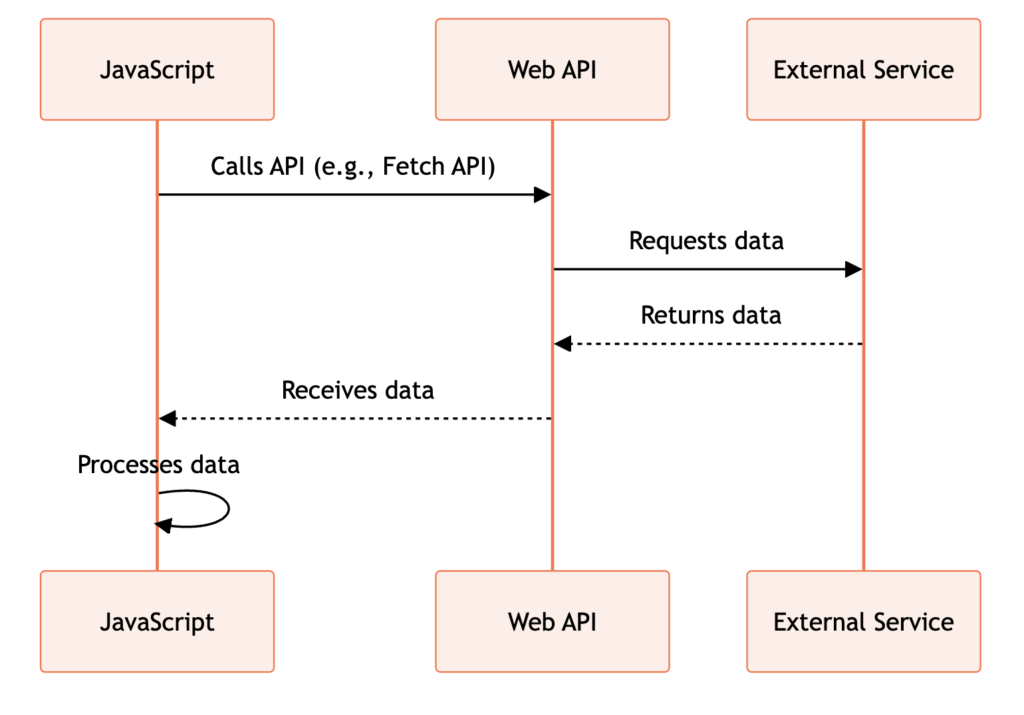
Libraries and Frameworks
JavaScript has a vibrant ecosystem with a wide range of libraries and frameworks that can significantly simplify and enhance your development process. Let’s explore some popular JavaScript libraries and frameworks:
- Popular JavaScript Libraries: jQuery is one of the most widely used JavaScript libraries. It provides a concise and powerful API for DOM manipulation, event handling, AJAX, and more. With jQuery, developers can easily select elements, manipulate their properties and attributes, handle events, and make asynchronous requests to the server. It abstracts away many of the complexities of working with the DOM and provides a consistent and cross-browser compatible interface. Apart from jQuery, there are many other popular JavaScript libraries available, such as Lodash, Underscore.js, and Moment.js, which provide additional utility functions and features to simplify common programming tasks.
- Introduction to JavaScript Frameworks: JavaScript frameworks like React, Angular, and Vue.js provide a structured and efficient way to build complex web applications. They offer features like component-based architecture, virtual DOM, and state management. React, developed by Facebook, is a declarative and efficient library for building user interfaces. It allows developers to create reusable UI components and efficiently update only the necessary parts of the DOM when the state changes. Angular, developed by Google, is a full-featured framework that provides a complete solution for building large-scale applications. It offers features like two-way data binding, dependency injection, and a powerful templating system. Vue.js, on the other hand, is a lightweight framework that focuses on simplicity and ease of use. It provides a flexible and intuitive API for building reactive user interfaces. These frameworks have gained popularity due to their ability to improve developer productivity, promote code reusability, and provide efficient rendering and performance optimizations.
JavaScript in Web Development
JavaScript plays a crucial role in web development, both on the frontend and backend. Let’s explore its significance in these areas:
- Role of JavaScript in Frontend Development: JavaScript is primarily used to add interactivity and dynamic behavior to websites. It allows you to create engaging user interfaces, handle user input, and communicate with servers.
- JavaScript for Backend Development (Node.js): Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows you to run JavaScript on the server-side, enabling you to build scalable and efficient web applications.
JavaScript has revolutionized frontend development by providing the ability to create highly interactive and dynamic websites. With JavaScript, developers can manipulate the Document Object Model (DOM) of a webpage, allowing them to dynamically update content, respond to user actions, and create visually appealing effects.
JavaScript also enables developers to communicate with servers and retrieve data without the need for a page refresh. This is done through techniques like AJAX (Asynchronous JavaScript and XML) and Fetch API, which allow websites to make asynchronous requests to servers, retrieve data in the background, and update the webpage dynamically without interrupting the user’s interaction.
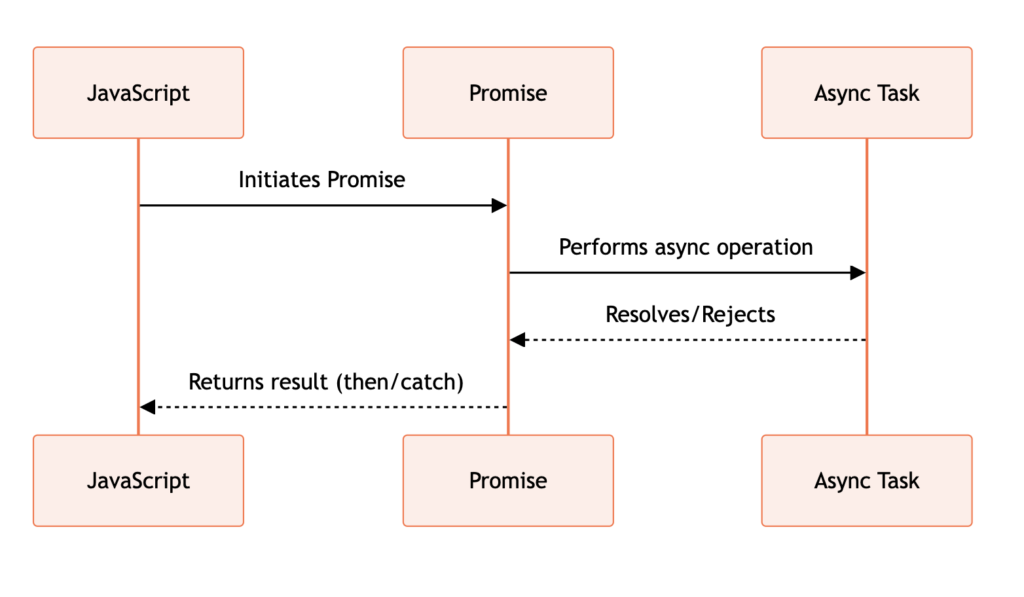
On the backend, JavaScript is no longer limited to just the frontend. With the introduction of Node.js, developers can now use JavaScript to build server-side applications. Node.js is a powerful runtime environment that allows JavaScript to run on the server, providing a unified language for both frontend and backend development.
Node.js utilizes an event-driven, non-blocking I/O model, which makes it highly scalable and efficient. It is particularly well-suited for building real-time applications, chat servers, streaming services, and other applications that require high concurrency and low latency.
Furthermore, Node.js has a vast ecosystem of libraries and frameworks that make backend development with JavaScript even more productive and efficient. Express.js, for example, is a popular web application framework that simplifies the process of building robust and scalable server-side applications.
Advanced JavaScript Concepts
Once you have a solid understanding of the basics, you can explore some advanced JavaScript concepts. Let’s take a look at a couple of them:
- Asynchronous JavaScript: Asynchronous programming is a key aspect of JavaScript. It allows you to perform tasks concurrently, without blocking the execution of other code. JavaScript provides various techniques like callbacks, promises, and async/await to handle asynchronous operations.
- ES6 Features and Beyond: ES6 (ECMAScript 2015) introduced several new features and enhancements to JavaScript. These include arrow functions, template literals, destructuring assignments, classes, modules, and more. Subsequent versions of ECMAScript have also introduced additional features.
- Functional Programming: JavaScript supports functional programming paradigms, which can make your code more concise, modular, and easier to reason about. Functional programming emphasizes immutability, pure functions, and higher-order functions. It also provides powerful array methods like map, filter, and reduce, which allow you to perform complex operations on arrays in a declarative manner.
- Design Patterns: Design patterns are reusable solutions to common problems in software design. JavaScript developers often use design patterns to structure their code and improve its maintainability and reusability. Some popular design patterns in JavaScript include the Singleton pattern, Observer pattern, and Module pattern.
- Performance Optimization: As your JavaScript applications grow in complexity, it becomes important to optimize their performance. This involves techniques like minimizing network requests, caching data, lazy loading, and using algorithms with better time complexity. JavaScript tools like performance profilers can help you identify bottlenecks and optimize your code.
By diving into these advanced concepts, you can take your JavaScript skills to the next level and build more robust and efficient applications.
These are just a few reasons why learning JavaScript is beneficial. Whether you are a beginner or an experienced developer, adding JavaScript to your skillset can open up a world of opportunities and help you stay ahead in the rapidly changing tech landscape.
Would you like to get engaged with professional Specialists?
We are a software development team with extensive development experience in the Hybrid and Crossplatform Applications development space. Let’s discuss your needs and requirements to find your best fit.
Getting Started with JavaScript
If you are ready to dive into JavaScript and start your learning journey, here are some resources to help you get started:
Resources for Learning JavaScript: There are numerous online tutorials, courses, and books available to learn JavaScript. Some popular resources include MDN Web Docs, freeCodeCamp, Codecademy, and Eloquent JavaScript.
Best Free JavaScript Learning Sources
Source Name | Description |
---|---|
Mozilla Developer Network (MDN) | A comprehensive resource that offers detailed documentation, tutorials, and guides on JavaScript and all web technologies. |
JavaScript.info | Provides a free modern JavaScript tutorial from the basics to advanced topics, with simple explanations and exercises. |
Codecademy | An interactive platform that teaches JavaScript programming fundamentals along with HTML and CSS integration. |
Khan Academy | Offers practice exercises and instructional videos on JavaScript programming and other computer science topics. |
freeCodeCamp | This community-driven website helps you learn to code by building projects and earning certifications. |
Coursera | Provides various courses on JavaScript, including options to learn for free (audit only). |
Eloquent JavaScript | A book providing an insightful overview of JavaScript, which is also available online for free. |
Udemy | Udemy offers a range of free courses in JavaScript that include video lectures and exercises. |
edX | Access to a selection of JavaScript courses from universities and colleges around the world, some of which are free. |
SoloLearn | This is a mobile-friendly platform for learning JavaScript from scratch, offering a free course with an interactive community. |
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
Looking ahead, the future of JavaScript looks promising. The ECMAScript committee is constantly working on new proposals and additions to the language, with ECMAScript 9, or ES9/ES2018, already being released. ES9 introduced features like asynchronous iteration, rest/spread properties, and additional regular expression features.
Now that you have an understanding of the resources available for learning JavaScript, some tips for beginners, and how to set up your development environment, you are ready to start your JavaScript journey. Remember, learning a programming language takes time and practice, so be patient and persistent. With dedication and consistent effort, you’ll soon be writing JavaScript code like a pro!