Unveiling C#
C# programming, also known as C Sharp, is a versatile and powerful language that holds both strengths and weaknesses. Understanding the practical applications of C# can provide developers with the tools to create efficient and robust software solutions. Additionally, creating a to-do app using C# offers a hands-on opportunity to comprehend the language’s capabilities in real-world scenarios.
Exploring the strengths and weaknesses of C# programming allows developers to grasp its potential while being aware of its limitations. This understanding is crucial for leveraging the language effectively in software development projects. Moreover, the process of creating a to-do app using C# exemplifies how this programming language can be applied to develop practical and user-friendly applications.
By embracing C#, developers can harness its strengths while being mindful of its limitations, ultimately leading to proficient and strategic utilization in their projects.
The Power of C#
Intuitive Syntax and Powerful Features
C# programming language is renowned for its intuitive syntax, which allows developers to write code that is clear, concise, and easy to understand. The syntax of C# is designed to resemble spoken language, making it accessible for both beginners and experienced programmers. This intuitive nature enables developers to grasp C# programming concepts more efficiently, leading to faster development cycles and reduced chances of errors.
In addition to its intuitive syntax, C# boasts a wide array of powerful features that contribute to its versatility and effectiveness in software development. These features include robust type-safety, automatic garbage collection, and seamless integration with the .NET framework. The combination of these features empowers developers to create complex applications while maintaining code integrity and security.
Practical Applications
The practical applications of C# programming span across various industries and domains. From web development to mobile app creation, C# is utilized in a multitude of real-world scenarios. In web development, C# is often employed for backend services and server-side scripting due to its efficiency and scalability. Furthermore, C# plays a pivotal role in the development of desktop applications for Windows operating systems.
Moreover, C# is extensively used in software development for creating enterprise-level solutions such as customer relationship management (CRM) systems, inventory management software, and financial analysis tools. Its ability to seamlessly integrate with databases and cloud services makes it an ideal choice for building robust business applications.
By comprehending the practical applications of C#, developers can leverage its capabilities across diverse domains, contributing to the creation of innovative and impactful software solutions.
Creating a To-Do App in C#
Process of Creating a To-Do App
Creating a to-do app using C# involves a systematic process that allows developers to harness the language’s capabilities in building efficient task management applications. Here is a step-by-step guide to creating a to-do app using C#:
-
Planning and Design:
-
The initial phase involves outlining the features and functionalities of the to-do app. This includes defining the user interface, task management components, and any additional features such as notifications or reminders.
-
-
Setting Up the Development Environment: Developers need to set up their integrated development environment (IDE) for C# programming. Visual Studio, a popular IDE, provides a comprehensive set of tools for developing C# applications.
-
Coding the User Interface: Using Windows Presentation Foundation (WPF) or Windows Forms, developers can design the visual elements of the to-do app. This includes creating interactive task lists, input fields for adding new tasks, and options for marking tasks as complete.
-
Implementing Task Management Logic: The core functionality of the to-do app revolves around managing tasks. Developers use C# to implement logic for adding tasks, updating task statuses, and removing completed tasks from the list.
Task Class:
public class Task
{
public string Name { get; set; }
public string Description { get; set; }
public bool IsCompleted { get; set; }
public Task(string name, string description = "")
{
Name = name;
Description = description;
IsCompleted = false;
}
}
Explanation:
- Defines a
Task
class to represent individual tasks. - It has properties for:
Name
: The task’s name.Description
: Optional details about the task.IsCompleted
: A boolean flag indicating whether the task is done.
- The constructor allows creating task objects with a name and optional description.
Data Structure:
private List<Task> tasks = new List<Task>();
Explanation:
- Creates a
List<Task>
object to store tasks in memory. - This acts as the app’s primary data storage for managing tasks.
Adding a Task:
private void AddTaskButton_Click(object sender, EventArgs e)
{
string taskName = TaskNameTextBox.Text;
Task newTask = new Task(taskName);
tasks.Add(newTask);
TaskListBox.Items.Add(taskName); // Assuming a ListBox for display
TaskNameTextBox.Clear(); // Clear input field
}
Here are code snippets for key functionalities, accompanied by explanations:
1. Task Class:
public class Task
{
public string Name { get; set; }
public string Description { get; set; }
public bool IsCompleted { get; set; }
public Task(string name, string description = "")
{
Name = name;
Description = description;
IsCompleted = false;
}
}
Explanation:
- Defines a
Task
class to represent individual tasks. - It has properties for:
Name
: The task’s name.Description
: Optional details about the task.IsCompleted
: A boolean flag indicating whether the task is done.
- The constructor allows creating task objects with a name and optional description.
2. Data Structure:
private List<Task> tasks = new List<Task>();
Explanation:
- Creates a
List<Task>
object to store tasks in memory. - This acts as the app’s primary data storage for managing tasks.
3. Adding a Task:
private void AddTaskButton_Click(object sender, EventArgs e)
{
string taskName = TaskNameTextBox.Text;
Task newTask = new Task(taskName);
tasks.Add(newTask);
TaskListBox.Items.Add(taskName); // Assuming a ListBox for display
TaskNameTextBox.Clear(); // Clear input field
}
Explanation:
- This code handles a button click event for adding tasks.
- It retrieves the task name from a text box.
- Creates a new
Task
object with that name. - Adds the task to the
tasks
list. - Updates the UI (assuming a ListBox) to display the new task.
- Clears the input field for the next task.
Marking a Task Complete:
private void TaskListBox_SelectedIndexChanged(object sender, EventArgs e)
{
int selectedIndex = TaskListBox.SelectedIndex;
if (selectedIndex >= 0)
{
Task selectedTask = tasks[selectedIndex];
selectedTask.IsCompleted = !selectedTask.IsCompleted;
TaskListBox.Items[selectedIndex] = FormatTaskDisplay(selectedTask); // Update UI
}
}
Explanation:
- This code handles selection changes in the task list (assuming a ListBox).
- It retrieves the selected task index.
- If a task is selected, it toggles its
IsCompleted
property. - Updates the UI to reflect the new completion status (e.g., strikethrough or different color).
Saving Tasks to a Database:
private void SaveTasksToDatabase()
{
using (SQLiteConnection connection = new SQLiteConnection("path/to/database.db"))
{
connection.Open();
SQLiteCommand command = new SQLiteCommand("INSERT INTO Tasks (Name, Description, IsCompleted) VALUES (@Name, @Description, @IsCompleted)", connection);
foreach (Task task in tasks)
{
command.Parameters.Clear();
command.Parameters.AddWithValue("@Name", task.Name);
command.Parameters.AddWithValue("@Description", task.Description);
command.Parameters.AddWithValue("@IsCompleted", task.IsCompleted);
command.ExecuteNonQuery();
}
}
}
Explanation:
- This code demonstrates saving tasks to an SQLite database.
- It establishes a connection to the database.
- Prepares an SQL INSERT command to add tasks.
- Iterates through the
tasks
list and inserts each task into the database.
Data Persistence, Testing & Debugging and finally Deploying your C# App
- Incorporating Data Persistence: To ensure that tasks are saved even when the application is closed, developers utilize database technologies such as SQL Server or SQLite with C# to store task data securely.
- Testing and Debugging: Thorough testing is essential to identify and rectify any issues within the application. Through debugging in Visual Studio or other debugging tools, developers can ensure that the app functions seamlessly.
- Deployment and Distribution: Once the app is thoroughly tested and debugged, it can be deployed on various platforms such as Windows Store or distributed as an executable file for users to download and install.
By following these steps, developers can create a robust and user-friendly to-do app using C#, showcasing how this programming language can be effectively utilized in practical applications.
Benefits of a C# To-Do App
A to-do app developed in C# offers several advantages that contribute to enhanced productivity and streamlined task management:
- Efficient Task Organization: The intuitive nature of C# allows developers to create an organized and user-friendly interface for managing tasks efficiently.
- Seamless Integration with Other Tools: C# facilitates seamless integration with other Microsoft technologies such as Azure DevOps or Microsoft Office Suite, enabling users to synchronize their tasks across multiple platforms effortlessly.
- Scalability and Customization: With C#, developers can build scalable apps that can accommodate future feature enhancements while also offering customization options tailored to specific user needs.
- Enhanced Security Features: Leveraging C#’s robust security features ensures that sensitive task data remains secure within the application.
By harnessing these benefits, a C# to-do app not only simplifies task management but also showcases the versatility of this programming language in developing practical and impactful applications.
Advantages of Learning C#
Versatility and Flexibility
When it comes to the advantages of learning C# programming, one cannot overlook its remarkable versatility and flexibility. C# offers a wide range of applications across various domains, making it an ideal choice for developers seeking a language with diverse use cases.
In application development, C# showcases its versatility by enabling developers to create solutions for desktop, web, mobile, cloud-based, and enterprise-level platforms. This adaptability allows programmers to apply their skills to a multitude of projects, from creating interactive user interfaces for desktop applications to developing scalable web services.
Moreover, the flexibility of C# programming lies in its ability to seamlessly integrate with other technologies and frameworks. Whether it’s integrating with databases using Entity Framework or collaborating with front-end technologies like Angular or React, C# provides developers with the flexibility to work across different tech stacks while maintaining code integrity and efficiency.
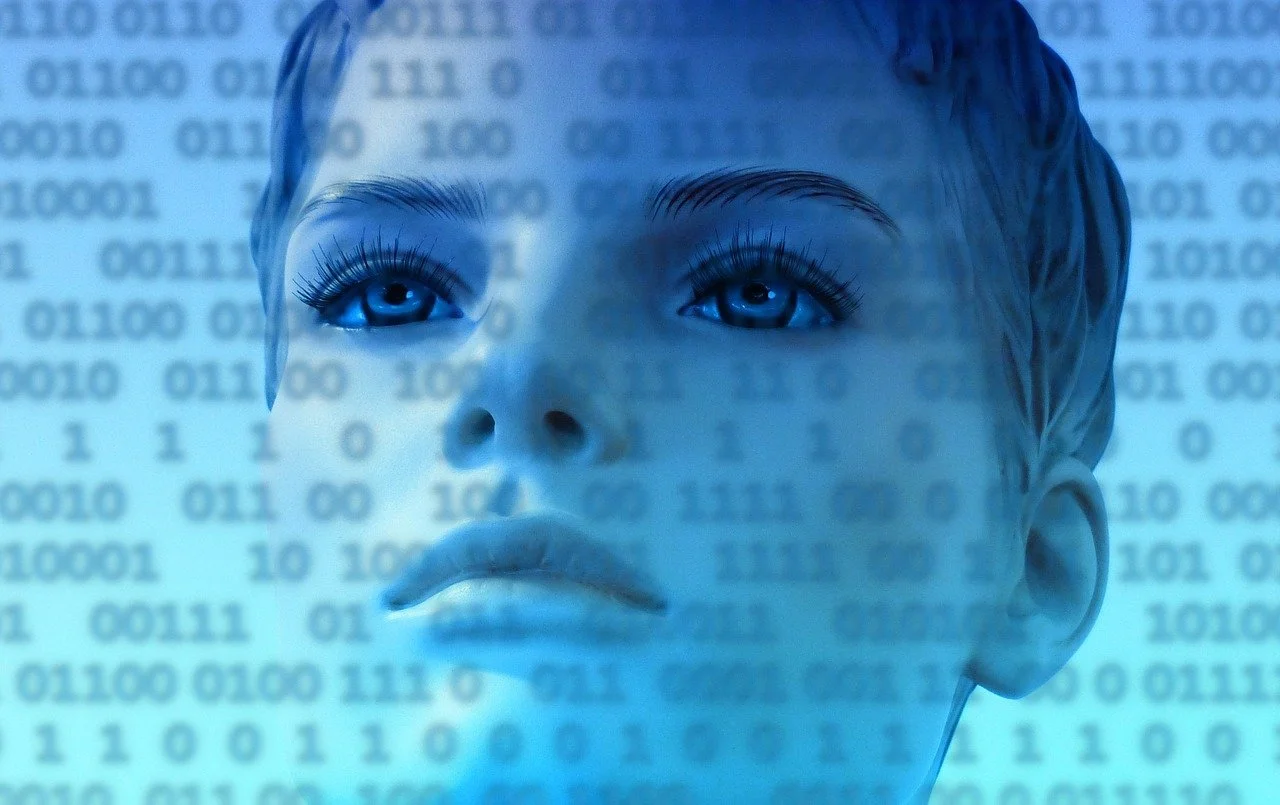
C# in a nutshell
C#‘s versatility and flexibility empower developers to tackle diverse projects and adapt to evolving technological landscapes effortlessly.
Career Opportunities
The demand for C# developers in the job market is substantial and continues to grow as more industries recognize the value of this programming language. With proficiency in C#, programmers can explore a myriad of career prospects and growth opportunities across various sectors.
In the software development industry, companies actively seek skilled C# developers for roles such as software engineers, application developers, systems analysts, and technical leads. The proficiency in C# opens doors to opportunities in both established enterprises and innovative startups that rely on robust software solutions.
Furthermore, the career prospects for C# programmers extend beyond traditional software development roles. As technology continues to evolve, specialized domains such as cloud computing, cybersecurity, and artificial intelligence present exciting pathways for professionals proficient in C#. These domains offer lucrative career growth opportunities where knowledge of C# is highly valued.
By mastering C#, individuals can position themselves for a rewarding career journey filled with diverse opportunities for professional advancement and specialization.
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
Common Challenges in C#
C# Learning Curve
When delving into the realm of C# programming, beginners often encounter a set of obstacles that contribute to the learning curve. These difficulties in learning C# can pose challenges for individuals who are new to the language and its associated development environment. Understanding and addressing these challenges is essential for aspiring developers to navigate their learning journey effectively.
One of the primary difficulties in learning C# programming is grasping the concepts of object-oriented programming (OOP). Object-oriented programming forms the foundation of C#, and comprehending its principles, such as classes, objects, inheritance, and polymorphism, can be daunting for beginners. Additionally, understanding complex topics like asynchronous programming and LINQ (Language-Integrated Query) requires time and dedication.
To overcome these challenges, beginners can adopt various strategies to streamline their learning process. Engaging with comprehensive tutorials, online courses, and interactive coding exercises can provide a structured approach to mastering C#. Additionally, seeking mentorship or joining developer communities allows novices to benefit from the experience and guidance of seasoned professionals. Embracing a hands-on approach by working on small projects or contributing to open-source initiatives fosters practical application of theoretical knowledge.
Debugging and Troubleshooting C#
In the realm of C# development, debugging poses as one of the common challenges encountered by programmers. Identifying and resolving issues within code demands a systematic approach coupled with an understanding of debugging tools and techniques specific to C# programming.
Common debugging challenges in C# include pinpointing logical errors that lead to unexpected program behavior, identifying memory leaks that affect application performance, and troubleshooting issues related to asynchronous code execution. Moreover, handling exceptions effectively while maintaining code integrity adds another layer of complexity to the debugging process.
To address these challenges, developers can employ effective troubleshooting practices tailored to C# programming. Leveraging integrated development environments (IDEs) such as Visual Studio equips developers with robust debugging tools including breakpoints, watch windows, and step-by-step execution capabilities. Familiarizing oneself with logging frameworks for error tracking and utilizing unit testing for validating individual components contributes significantly to efficient troubleshooting in C# development.
By recognizing these common challenges in learning C# programming and honing effective debugging techniques, developers can enhance their proficiency in utilizing this powerful language for software development endeavors.
Industry Demand for C# Developers
Current Market Trends
The job market for C# programmers continues to exhibit robust demand, reflecting the language’s significance in the software development industry. Employers across diverse sectors actively seek professionals proficient in C# programming to drive innovation and develop cutting-edge solutions. The employment outlook for C# developers remains promising, with ample opportunities for career growth and specialization.
In the current software development landscape, the demand for C# skills is particularly pronounced in domains such as web application development, enterprise software solutions, and cloud-based services. Companies prioritize hiring developers with expertise in C# to spearhead projects that require scalable and secure applications. This trend underscores the pivotal role of C# in addressing contemporary technological challenges and fulfilling evolving business needs.
Furthermore, the employment outlook for C# developers extends beyond traditional software companies to encompass industries such as finance, healthcare, and e-commerce. Organizations recognize the adaptability of C# in creating tailored solutions that align with industry-specific requirements, thereby amplifying its relevance across diverse sectors.
As businesses continue to embrace digital transformation initiatives, the job market for C# programmers is poised for sustained growth. The language’s versatility and compatibility with emerging technologies position it as a cornerstone of modern software development practices.
Future Prospects
Predictions for the future demand for C# programmers indicate a trajectory of continued expansion driven by evolving industry dynamics. As technology becomes increasingly intertwined with daily operations across various sectors, the role of C# in the technology sector is set to evolve significantly.
The future prospects for C# programming encompass a spectrum of opportunities spanning emerging domains such as artificial intelligence (AI), machine learning, and data analytics. With its robust features and seamless integration capabilities, C# is primed to play a pivotal role in powering AI-driven applications and IoT (Internet of Things) ecosystems.
Moreover, as businesses strive to leverage data-driven insights and sophisticated automation technologies, the demand for skilled C# developers is expected to surge. The evolving role of C# in these transformative domains positions it as an indispensable asset for driving innovation and shaping the technological landscape.
In essence, the future prospects for C# programming are characterized by an amalgamation of traditional software development roles with emerging frontiers such as AI and IoT. Professionals adept in C# are well-positioned to capitalize on these future opportunities while contributing to groundbreaking advancements at the intersection of technology and business.
The Future of C#
Emerging Technologies
As the technological landscape continues to evolve, the role of C# in emerging technologies such as AI and IoT is poised for significant expansion. The future advancements and applications of C# programming are set to revolutionize various industries, driving innovation and shaping the next generation of software solutions.
In the realm of artificial intelligence (AI), C# is positioned to play a pivotal role in powering intelligent applications that leverage machine learning algorithms, natural language processing, and predictive analytics. The robust features of C#, including its intuitive syntax and seamless integration with data science frameworks, make it an ideal choice for developing AI-driven solutions. From intelligent chatbots to sophisticated recommendation systems, C# empowers developers to create AI applications that redefine user experiences and drive operational efficiencies.
Moreover, in the domain of Internet of Things (IoT), C# offers a compelling framework for building interconnected ecosystems that encompass smart devices, sensors, and cloud-based services. The future prospects of C# programming in IoT entail enabling developers to craft scalable and secure IoT solutions that cater to diverse industry verticals. By harnessing C#’s versatility and flexibility, IoT applications built with C# can facilitate seamless data exchange, real-time monitoring, and advanced automation across interconnected devices.
The convergence of C# with emerging technologies represents a paradigm shift in software development paradigms. By embracing these advancements, developers can unlock new frontiers in creating innovative solutions that address complex challenges while enhancing user experiences.
Integration with Other Languages
One of the remarkable attributes of C# programming lies in its seamless integration with other programming languages, offering collaborative opportunities and cross-language compatibility. The interoperability of C# enables developers to leverage existing codebases written in languages such as C++, Python, or Java while incorporating them into C# projects seamlessly.
C#’s compatibility with languages like JavaScript facilitates the development of full-stack web applications by enabling server-side scripting using ASP.NET alongside client-side scripting powered by JavaScript frameworks. This collaborative synergy between languages empowers developers to build dynamic web experiences while leveraging their expertise across multiple tech stacks.
Furthermore, through interoperability with native code written in languages like C or C++, developers can optimize performance-critical components within their applications by integrating them into their C# projects. This cross-language compatibility enhances the extensibility and performance capabilities of software solutions developed using C#, opening doors to diverse collaboration opportunities within the developer community.
In essence, the integration capabilities of C# foster a collaborative ecosystem where developers can harness their proficiency across multiple languages to create comprehensive and innovative software solutions that transcend traditional boundaries.
Best Practices
Coding Standards
Adhering to coding standards is paramount in effective C# programming. By following established coding conventions and best practices, developers can ensure that their code is not only clean and readable but also efficient and maintainable. Consistency in coding standards across projects and teams fosters collaboration and enhances the overall quality of software solutions.
Some best practices for maintaining coding standards in C# include:
- Naming Conventions: Utilize meaningful and descriptive names for variables, methods, classes, and other elements within the codebase. This promotes clarity and comprehension, aiding both developers and maintainers in understanding the purpose of each component.
- Indentation and Formatting: Consistently apply indentation rules and formatting guidelines to enhance the visual structure of the code. This includes using appropriate spacing, line breaks, and alignment to improve readability.
- Comments and Documentation: Incorporate clear and concise comments to elucidate complex logic or provide context for specific code segments. Additionally, maintain up-to-date documentation to outline the functionality of classes, methods, and interfaces.
Furthermore, leveraging static code analysis tools such as ReSharper or StyleCop can assist in enforcing coding standards throughout the development process. These tools identify deviations from established conventions and offer suggestions for aligning the code with best practices.
By upholding coding standards in C# development, programmers can elevate the quality of their codebase while fostering a collaborative environment conducive to effective software development.
Continuous Learning
Continuous learning is a fundamental aspect of mastering C# programming. As technology evolves at a rapid pace, staying updated with emerging trends, best practices, and advancements in C# is essential for professional growth.
To engage in continuous learning effectively:
- Explore Online Resources: Leverage online platforms such as Pluralsight, Udemy, or Coursera to access courses covering advanced C# concepts, design patterns, performance optimization techniques, and more.
- Participate in Developer Communities: Join forums like Stack Overflow or GitHub discussions to seek insights from experienced developers on complex topics or troubleshooting challenges encountered during development.
- Read Industry Publications: Stay informed about industry trends by reading blogs, articles, whitepapers, and publications related to C# programming advancements.
- Contribute to Open Source Projects: Engage with open-source initiatives on platforms like GitHub to collaborate with peers on real-world projects while honing practical skills.
Moreover, attending conferences or webinars focused on C# programming provides opportunities for networking with industry experts while gaining exposure to innovative concepts shaping the future of software development.
Embracing continuous learning equips developers with the knowledge and skills required to excel in C# programming while adapting seamlessly to evolving technological landscapes.
Embracing C#
As we conclude our exploration of C# programming, it becomes evident that understanding this versatile language opens doors to a multitude of opportunities. Grasping C# programming allows developers to delve into the realm of software development with confidence and proficiency. By comprehending the intricacies of the C# language, individuals can harness its power to create innovative solutions and contribute to technological advancements.
Looking ahead, the future of C# development presents exciting prospects for professionals seeking to carve a successful career path. The evolving landscape of technology offers abundant opportunities for those proficient in C#, ranging from traditional software development roles to specialized domains such as AI, IoT, and data analytics.
Embracing C# programming is paramount for aspiring developers and seasoned professionals alike. The importance of embracing C# lies in its potential to shape career growth by providing access to diverse and dynamic roles within the software development ecosystem. As businesses continue to prioritize digital transformation initiatives, the demand for skilled C# developers is set to soar, making it an opportune time to immerse oneself in this powerful programming language.
Embracing C# programming not only gives you the skills for success but also puts you at the forefront of innovation in the fast-changing tech world.