Python has emerged as one of the most popular and versatile programming languages in recent years. With its simplicity, readability, and extensive libraries, Python has become a go-to language for developers across various industries. In this article, we will explore the state of Python programming language in 2024, why it is important to learn Python, the areas in software development that can be covered by knowing Python, the evolution of the language, its utilization today, and how to get started with Python.
Why is it Important to Learn Python?
Python’s popularity stems from its ease of use and readability. Its syntax resembles plain English, making it accessible even to those with no prior programming experience. Learning Python opens up a world of opportunities as it is widely used in various domains, including web development, data analysis, artificial intelligence, machine learning, and automation. By mastering Python, you gain a valuable skill set that is highly sought after by employers in today’s technology-driven world.
Areas in Software Development Covered by Python
Python’s versatility allows it to be used in a wide range of software development areas. Here are a few key areas where Python excels:
- Web Development: Python offers powerful frameworks like Django and Flask, enabling developers to build robust and scalable web applications.
- Data Analysis and Visualization: Python’s extensive libraries, such as NumPy, Pandas, and Matplotlib, make it a popular choice for data analysis and visualization tasks.
- Machine Learning and Artificial Intelligence: Python’s libraries like TensorFlow, Keras, and Scikit-learn provide a solid foundation for developing machine learning models and AI applications.
- Automation and Scripting: Python’s simplicity and cross-platform compatibility make it ideal for automating repetitive tasks and scripting.
The Evolution of Python:
Python was first created by Guido van Rossum in the late 1980s, with the intention of creating a language that emphasized code readability. Over the years, Python has evolved through various versions, with each release introducing new features and improvements. Python 3, released in 2008, brought significant changes and improvements over Python 2, including better Unicode support, enhanced performance, and a more consistent syntax. In 2024, Python continues to evolve, with regular updates and contributions from a vibrant community of developers.
Utilization of Python Today
Python’s versatility and ease of use have contributed to its widespread adoption across industries. Today, Python is used by tech giants like Google, Facebook, and Netflix, as well as in scientific research, finance, and government organizations. Its simplicity and extensive libraries make it a preferred choice for rapid prototyping, data analysis, and building scalable applications. Python’s popularity is further boosted by its active community, which constantly develops new libraries, frameworks, and tools to support various use cases.
Getting Started with Python:
To start learning Python, you first need to install it on your computer. Python is available for Windows, macOS, and Linux, and can be downloaded from the official Python website (python.org). The installation process is straightforward and well-documented, with detailed instructions provided for each operating system.
Once you have Python installed, there are numerous online platforms where you can learn and practice Python for free. Websites like Codecademy, Coursera, and SoloLearn offer interactive Python courses suitable for beginners. These platforms provide a structured learning experience, with hands-on exercises and quizzes to reinforce your understanding. Additionally, online coding platforms like Replit and Jupyter Notebook allow you to write and run Python code directly in your browser, eliminating the need for local installations.
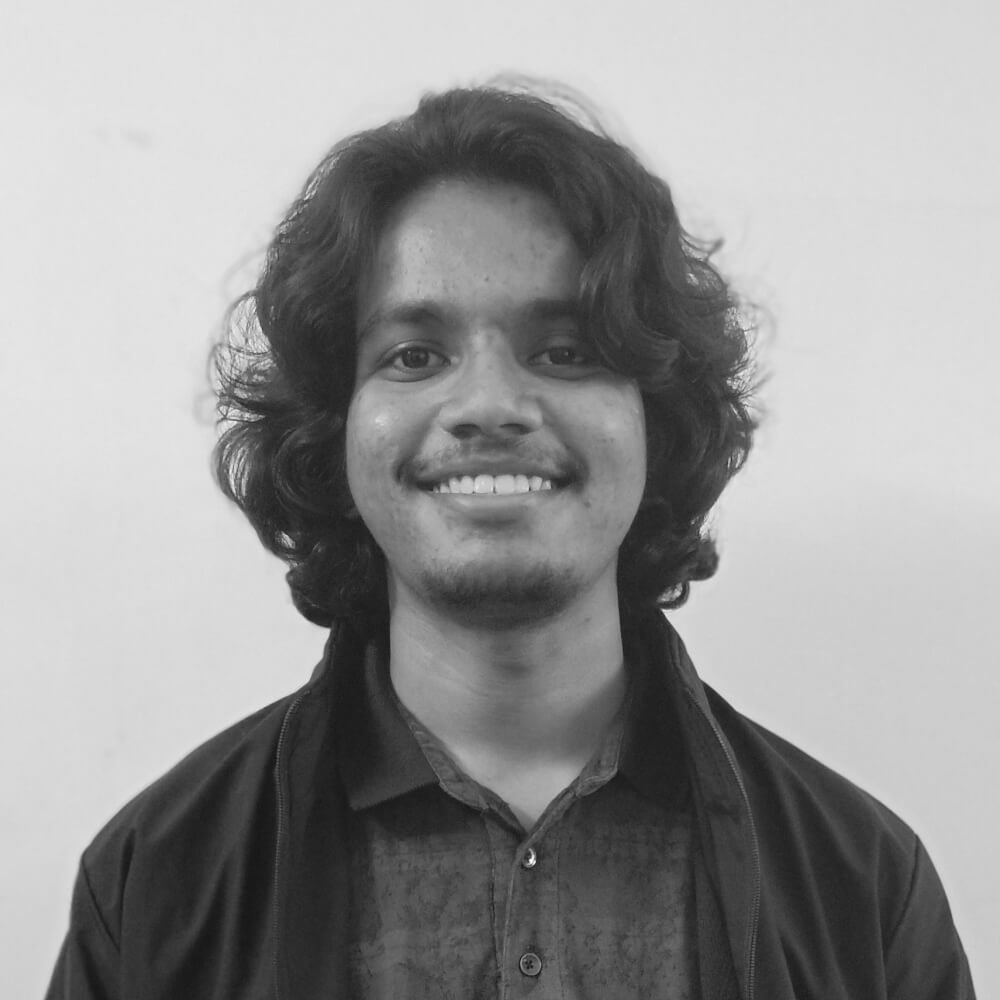
Learning Python in 2024 is more important than ever. Its versatility, simplicity, and extensive libraries make it an essential language for aspiring developers.
Whether you’re interested in web development, data analysis, machine learning, or automation, Python offers a wide range of possibilities. By investing time in learning Python and practicing through online platforms, you can gain a solid foundation in programming and open doors to exciting career opportunities in the tech industry.
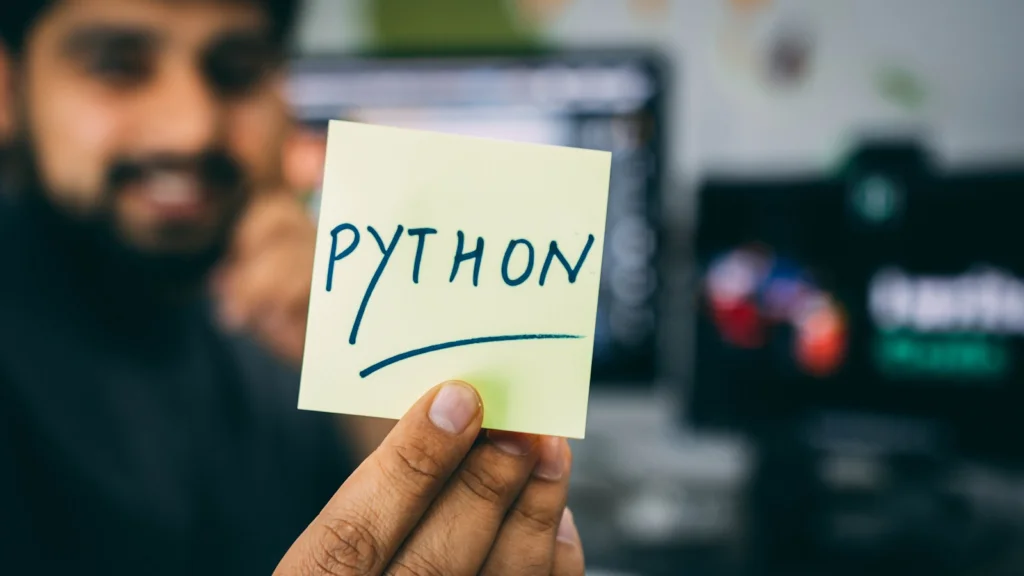
Building a To-Do List App with Python and Flask
Setup and Installation:
- Create a new project folder (e.g.,
my_todo_app
). - Set up a virtual environment:
mkdir my_todo_app
cd my_todo_app
python3 -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
3. Install Flask and Flask-SQLAlchemy:
pip install Flask Flask-SQLAlchemy
Create the Hello World App:
Create an app.py
file and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == "__main__":
app.run(debug=True)
Explanation:
- We create a Flask app and define a route for the root URL (
/
). - The
hello_world
function returns a simple greeting. - The
if __name__ == "__main__":
block ensures that the app runs only when executed directly (not when imported as a module).
Add a Database (SQLite):
We’ll use SQLAlchemy to manage our database. Add the following code to app.py
:
from flask import Flask, render_template
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///db.sqlite'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Todo(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
complete = db.Column(db.Boolean)
@app.route('/')
def home():
todo_list = Todo.query.all()
return render_template('base.html', todo_list=todo_list)
if __name__ == "__main__":
db.create_all()
app.run(debug=True)
Explanation:
- We configure our SQLite database using SQLAlchemy.
- The
Todo
model defines our to-do items with an ID, title, and completion status. - The
home
function queries all to-do items and renders an HTML template (base.html
).
Create the Template File:
Create a folder named templates
in your project root. Inside it, create a file named base.html
with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My To-Do List</title>
</head>
<body>
<h1>My To-Do List</h1>
<ul>
{% for todo in todo_list %}
<li>{{ todo.title }}</li>
{% endfor %}
</ul>
</body>
</html>
Explanation:
- We use Jinja2 templating to dynamically display the to-do items from the database.
Run the App:
Execute the following command in your terminal:
python app.py
Visit http://127.0.0.1:5000/ in your browser to see your running to-do app!
That’s it! You’ve built a basic to-do list app using Python and Flask.
Feel free to enhance it by adding features like task creation, deletion, and completion. 🚀🐍
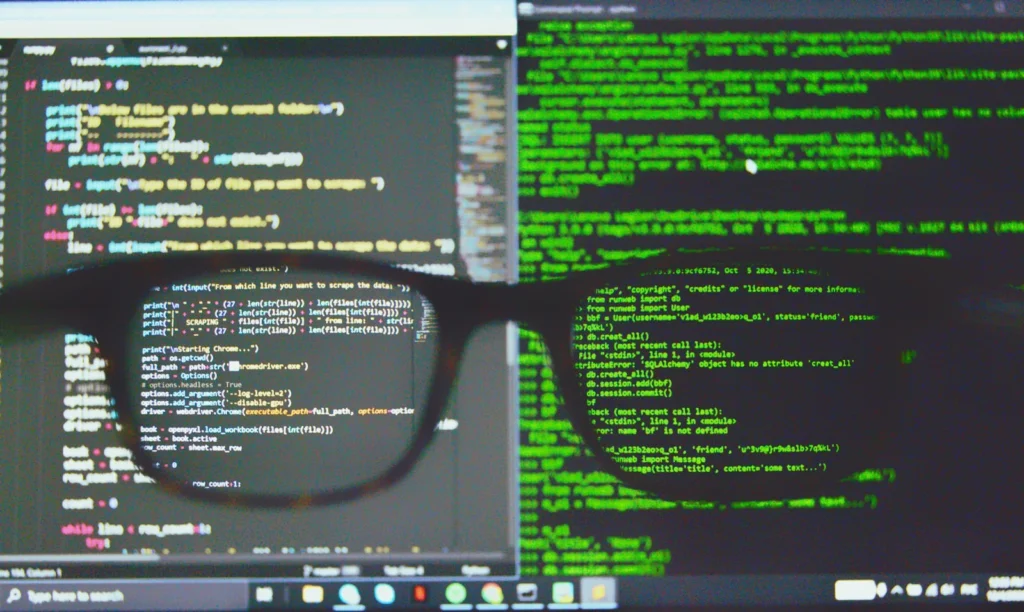
Where to Start Learning Python
Free Online Resources To Learn Python in 2024
Documentation
- Python official documentation: https://www.python.org/doc/
Courses
- freeCodeCamp Python course: https://www.freecodecamp.org/learn/scientific-computing-with-python/
- edX Python courses: https://www.edx.org/learn/python
YouTube
- Python for Beginners: A comprehensive tutorial
- Automating with Python: Practical projects
- Python for Data Science: An in-depth guide
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
The Best Free Online IDEs for Learning Python
Aspiring Python developers often seek accessible and user-friendly Integrated Development Environments (IDEs) to learn and practice their coding skills. Whether you’re a beginner or an experienced programmer, having the right tools can significantly enhance your learning experience. In this article, we’ll explore some of the best free online IDEs for learning Python, along with their features, pros, and cons.
LearnPython.org Workspace
Description:
- LearnPython.org Workspace is a cloud-based notebook that allows you to analyze data, collaborate, and share insights with your team.
- It’s suitable for both learning Python and performing actual data science work.
- The platform provides ready-to-use datasets, making it easy to practice and analyze real-world data.
- You can upload your own data and connect to your company’s data warehouse.
- Collaboration features allow you to edit and comment on projects, similar to Google Docs.
- Supports Python, R, and SQL.
Pros:
- Supports Python, R, and SQL.
- Offers built-in datasets and templates for quick coding start.
- Collaboration tools for sharing code.
- No installation required; works directly in your browser.
Cons:
- Requires an internet connection to code and access files.
JupyterLab and Jupyter Notebook
Description:
- JupyterLab and Jupyter Notebook are popular free notebook software for data science.
- Both are web-based tools that allow you to write code, view outputs, and add commentary using markdown.
- Jupyter Notebook has a simple document-centric interface, making it beginner-friendly.
- JupyterLab provides an interactive and modular development environment, allowing personalized workflows.
Pros:
- Supports workflows in data science, scientific computing, computational journalism, and machine learning.
- Beginner-friendly (Jupyter Notebook).
- Interactive and customizable (JupyterLab).
Cons:
- Requires an internet connection to access files.
Spyder
Description:
- Spyder is a lightweight, free, and open-source Python IDE.
- It’s excellent for data science and machine learning.
- Features interactive code execution, static code analysis, and integration with data science packages (NumPy, SciPy, Pandas, etc.).
- Includes a debugger and history log for command references.
Pros:
- Lightweight and efficient.
- Integrated with essential data science packages.
- Real-time code analysis.
- Interactive code execution.
Cons:
- Lacks project management capabilities.
Link: Spyder
Choose the IDE that best suits your learning style and project needs. Happy coding! 🐍🚀
Would you like to get engaged with professional Specialists?
We are a software development team with extensive development experience in the Hybrid and Crossplatform Applications development space. Let’s discuss your needs and requirements to find your best fit.