Selenium is a popular open-source framework used for automating web browsers. It enables testers and developers to write scripts in various programming languages to automate browser actions, such as clicking buttons, filling forms, and navigating through web pages. Selenium has become the go-to tool for test automation due to its flexibility, cross-browser compatibility, and extensive community support.
History and Evolution of the Selenium Project

The Selenium project was started by Jason Huggins in 2004 as an internal tool at ThoughtWorks. It was initially called “JavaScriptTestRunner” and was used to automate web applications for testing purposes. Later, it was renamed Selenium and released as an open-source project in 2004. Since then, it has gone through several iterations and enhancements, with the latest version being Selenium 4.
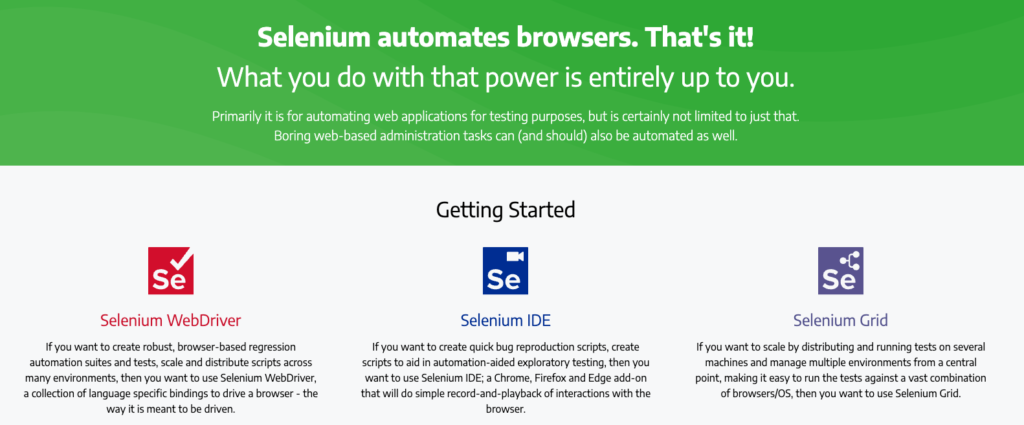
Selenium Components Explained
Selenium consists of three main components:
Selenium IDE
Selenium IDE (Integrated Development Environment) is a record and playback tool used for creating simple test cases. It is a browser extension that allows users to record their interactions with a web application and generate test scripts in various programming languages. Selenium IDE is a great tool for beginners and for quickly prototyping test cases.
Selenium WebDriver
Selenium WebDriver is the core component of Selenium. It provides a programming interface for interacting with web browsers. WebDriver allows users to write more complex and robust test scripts in programming languages like Java, Python, C#, and more. It supports multiple browsers, including Chrome, Firefox, Safari, and Edge, making it highly versatile.
Selenium Grid
Selenium Grid is a tool used for parallel test execution. It allows users to distribute test scripts across multiple machines and browsers, enabling faster execution and scalability. With Selenium Grid, you can run tests in parallel on different operating systems and browsers, reducing the overall test execution time.
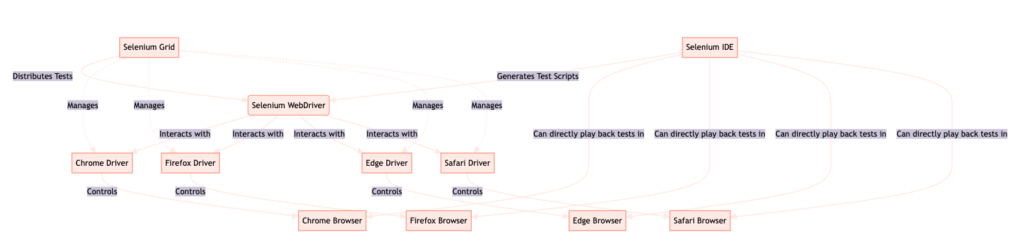
Comparing Selenium Components and When to Use Each
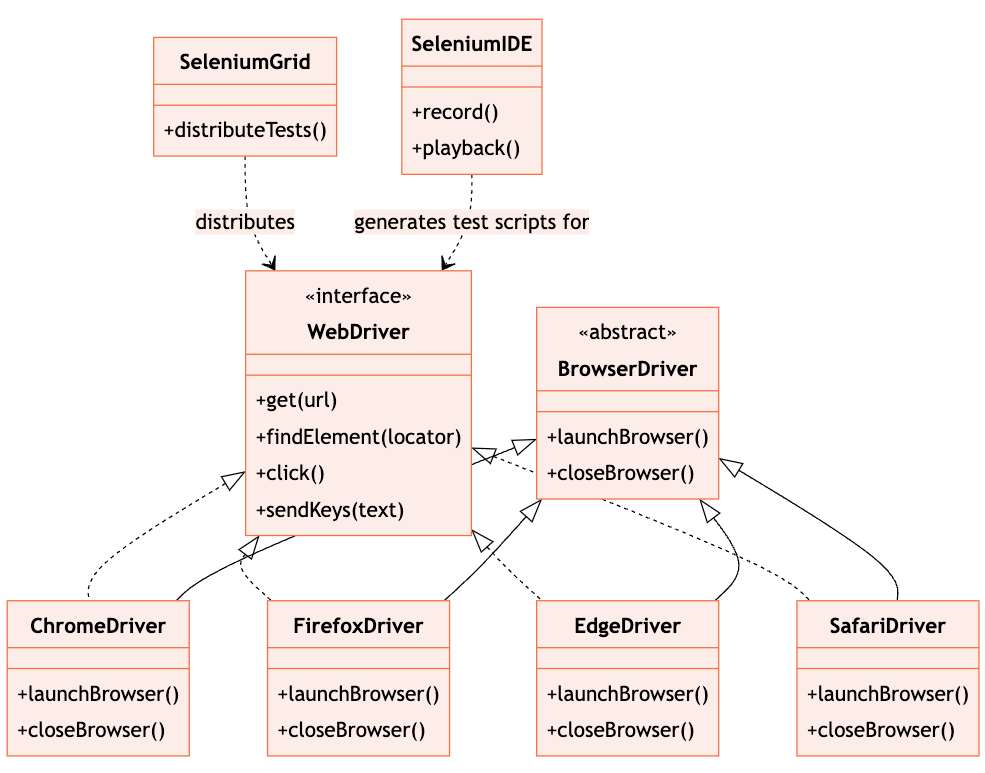
Setting Up Your Environment for Selenium
Before you can start writing Selenium test scripts, you need to set up your environment. Here is a step-by-step guide to installing and configuring Selenium:
Step 1: Install Java Development Kit (JDK)
Selenium requires Java to run. Install the latest version of JDK from the official Oracle website and set up the necessary environment variables.
Step 2: Choose a Programming Language
Decide on the programming language you want to use with Selenium. Popular choices include Java, Python, C#, and Ruby. Install the necessary development tools and libraries for your chosen language.
Step 3: Download Selenium WebDriver
Visit the official Selenium website and download the WebDriver for your preferred programming language. Add the WebDriver executable to your project’s dependencies or system PATH.
Step 4: Set Up a Development Environment
Set up a development environment for your chosen programming language. This may involve installing an Integrated Development Environment (IDE) or a code editor.
Step 5: Configure Selenium with Different Browsers
Depending on the browsers you want to automate, you may need to download and configure additional drivers. For example, Chrome requires ChromeDriver, Firefox requires GeckoDriver, and so on. Download the appropriate drivers and add them to your project’s dependencies or system PATH.
Writing Your First Selenium Test Script
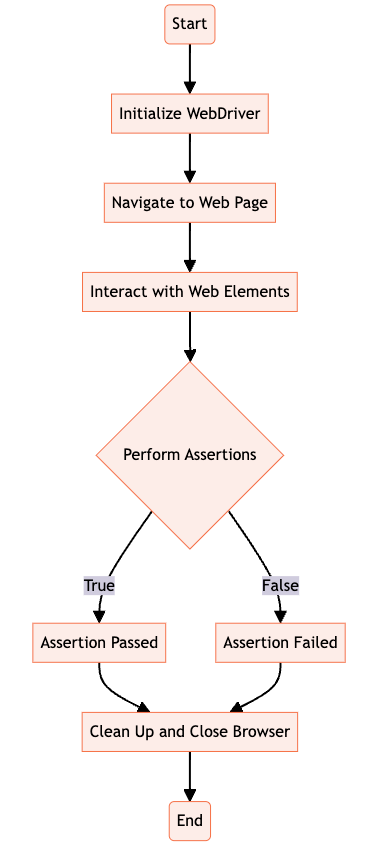
Now that your environment is set up, you can start writing Selenium test scripts. Here are the basics of writing a Selenium test script:
Step 1: Set Up a WebDriver Instance
Initialize a WebDriver instance for the browser you want to automate. This can be done by creating an object of the WebDriver class and specifying the browser type.
Step 2: Navigate to a Web Page
Use the WebDriver instance to navigate to a specific URL or web page. This can be done using the “get” method and providing the URL as a parameter.
Step 3: Interact with Web Elements
Use various Selenium commands and locators to interact with web elements on the page. Commands like “click”, “sendKeys”, and “submit” can be used to perform actions on buttons, input fields, and forms.
Step 4: Perform Assertions
Use assertions to verify that the expected behavior of the web application is met. Assertions can be used to check if specific elements are present, if certain text is displayed, or if certain conditions are true.
Step 5: Clean Up and Close the Browser
After the test script has completed, make sure to clean up any resources and close the browser. This can be done by calling the “quit” method on the WebDriver instance.
Basics of Writing Selenium Test Scripts
When writing Selenium test scripts, there are a few key concepts to keep in mind:
Selenium Commands
Selenium provides a set of commands that can be used to interact with web elements and perform actions. These commands include “click”, “sendKeys”, “submit”, “getText”, and many more. Familiarize yourself with the available commands and their usage.
Selenium Locators
Selenium uses locators to identify web elements on a page. Locators can be based on element IDs, class names, CSS selectors, XPath expressions, and more. Choose the most appropriate locator strategy based on the structure and attributes of the web page.
Advanced Selenium Scripting Techniques
As you become more proficient with Selenium, you can explore advanced scripting techniques. This includes handling dynamic elements, waiting for page loads and AJAX requests, working with frames and iframes, and handling pop-ups and alerts.
Implementing Page Object Model (POM) for Test Maintenance
The Page Object Model (POM) is a design pattern that helps in maintaining and organizing Selenium test scripts. It involves creating separate classes for each web page or component, encapsulating the page-specific elements and actions within these classes. This approach improves code reusability, readability, and maintainability.
Data-Driven Testing with Selenium
Data-driven testing is a technique where test cases are executed with different sets of test data. This approach allows for testing multiple scenarios and validating the behavior of the application under different conditions. Selenium supports data-driven testing by integrating with various data sources, such as Excel files, CSV files, databases, or external APIs.
Integrating Selenium with Testing Frameworks
Selenium can be easily integrated with popular testing frameworks like JUnit and TestNG. These frameworks provide additional features and functionalities for organizing and executing test cases. They also offer built-in reporting and assertion capabilities, making it easier to manage and analyze test results.
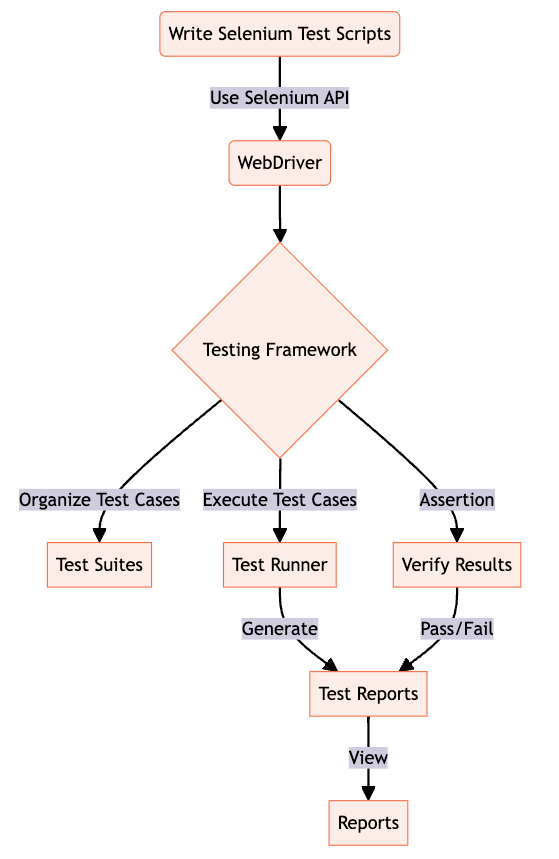
Best Practices for Structuring Test Cases
When structuring test cases in Selenium, it is important to follow best practices to ensure maintainability and reusability:
– Keep test cases modular and independent. Each test case should focus on a specific functionality or scenario.
– Use descriptive and meaningful names for test cases and test methods. This improves readability and makes it easier to understand the purpose of each test.
– Use comments to provide additional context and explanations for complex or critical test cases.
– Group related test cases into test suites or test classes. This allows for easier management and execution of test cases.
Debugging and Troubleshooting Selenium Tests
Despite careful scripting, issues and errors can occur during the execution of Selenium tests. Here are some common issues and how to resolve them:
– Element not found: This error occurs when the WebDriver is unable to locate a web element. Double-check the locator strategy and ensure that the element is present on the page.
– Timing issues: Selenium tests can sometimes fail due to timing issues, such as elements not being visible or clickable. Use explicit waits to wait for specific conditions before performing actions on elements.
– Browser compatibility: Different browsers may have slight variations in behavior and rendering. Test your scripts on multiple browsers to ensure cross-browser compatibility.
Continuous Integration and Deployment with Selenium
Continuous Integration (CI) and Continuous Deployment (CD) are practices that involve automating the build, testing, and deployment processes. Selenium can be integrated into CI/CD pipelines to ensure that tests are executed automatically whenever changes are made to the codebase. This helps in catching issues early and ensuring the stability of the application.
Setting Up Selenium Tests in CI/CD Pipelines
To set up Selenium tests in CI/CD pipelines, follow these steps:
Step 1: Choose a CI/CD Tool
Select a CI/CD tool that supports Selenium integration. Popular choices include Jenkins, Travis CI, CircleCI, and GitLab CI/CD.
Step 2: Configure the CI/CD Pipeline
Set up the CI/CD pipeline to trigger the execution of Selenium tests whenever changes are pushed to the code repository. Configure the pipeline to install the necessary dependencies, set up the test environment, and execute the test scripts.
Step 3: Generate Test Reports
Configure the CI/CD pipeline to generate test reports after the execution of Selenium tests. These reports provide detailed information about the test results, including passed and failed tests, test duration, and any errors or exceptions encountered.
Would you like to get engaged with professional Specialists?
We are a software development team with extensive development experience in the Hybrid and Crossplatform Applications development space. Let’s discuss your needs and requirements to find your best fit.
Leveraging Selenium Grid for Parallel Testing
Selenium Grid allows for parallel test execution across multiple machines and browsers, enabling faster test execution and scalability. To leverage Selenium Grid for parallel testing, follow these steps:
Step 1: Set Up a Selenium Grid Hub
Set up a Selenium Grid Hub, which acts as a central point for distributing test execution. The hub manages the test requests and delegates them to available Selenium Grid nodes.
Step 2: Set Up Selenium Grid Nodes
Set up Selenium Grid nodes on different machines and browsers. Each node registers with the hub and makes itself available for test execution.
Step 3: Configure Test Execution
Configure your Selenium test scripts to run against the Selenium Grid hub. Specify the desired browser and platform configurations to distribute the tests across the available nodes.
Step 4: Execute Tests in Parallel
Run your Selenium tests and observe the parallel execution across multiple machines and browsers. Selenium Grid automatically distributes the tests and collects the results for analysis.
Selenium for Mobile Testing
In addition to web testing, Selenium can also be used for mobile testing. Selenium provides the WebDriver protocol for automating mobile browsers, and there are additional tools like Appium that extend Selenium’s capabilities for mobile test automation.
Introduction to Appium for Mobile Test Automation with Selenium
Appium is an open-source tool that enables mobile test automation using Selenium WebDriver. It provides a WebDriver-compatible API for automating mobile applications on both iOS and Android platforms. With Appium, you can write cross-platform tests using the same Selenium API and programming language bindings.
Writing Cross-Platform Tests for iOS and Android
With Appium and Selenium, you can write cross-platform tests that run on both iOS and Android devices. The same test script can be executed on different platforms without any modifications, making it easier to maintain and reuse test code.
Best Practices and Tips for Selenium Test Automation
Here are some best practices and tips to keep in mind when working with Selenium for test automation:
Keep your test scripts clean and maintainable. Use proper indentation, comments, and meaningful variable names to improve readability.
Use version control to manage your test scripts. This allows for easy collaboration, versioning, and rollback of changes.
Use data-driven testing to cover multiple scenarios and validate different inputs and outputs.
Regularly update your Selenium and browser drivers to ensure compatibility and access to the latest features and bug fixes.
Scaling Selenium Tests for Large Projects
As your test suite grows, it becomes important to scale your Selenium tests to handle the increased complexity and volume. Here are some strategies for scaling Selenium tests:
– Use modular and reusable test code. Break down your test cases into smaller, independent units that can be easily maintained and executed.
– Use parallel test execution to reduce the overall test execution time. Selenium Grid and CI/CD pipelines can help in distributing tests across multiple machines and browsers.
– Implement a robust test data management strategy. Use external data sources, such as databases or APIs, to manage test data and reduce reliance on hard-coded values.
Exploring the Future of Selenium and Test Automation
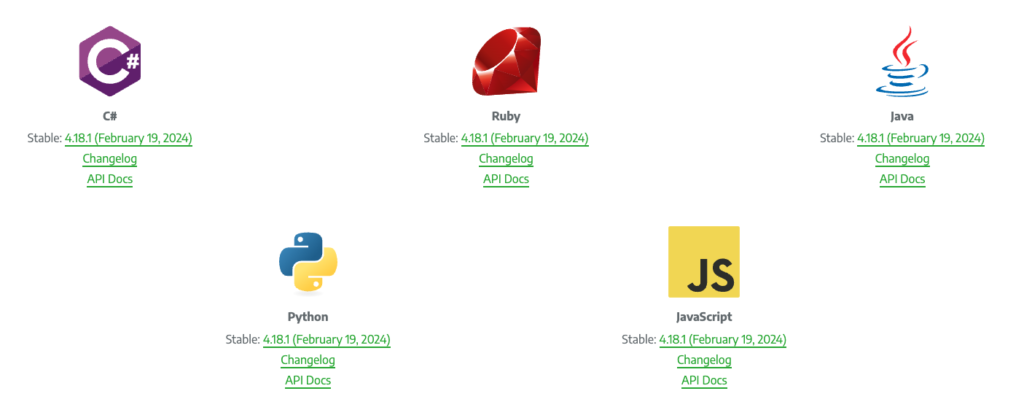
Selenium continues to evolve and adapt to the changing landscape of test automation. Here are some of the latest developments and emerging trends in Selenium and test automation:
Selenium 4: The latest version of Selenium, Selenium 4, introduces new features and improvements, including better support for mobile testing, enhanced browser support, and a more user-friendly API.
AI and Machine Learning: The integration of AI and machine learning technologies into test automation is gaining traction. These technologies can help in intelligent test case generation, test data generation, and test result analysis.
Headless Testing: Headless browsers, which run without a graphical user interface, are becoming more popular for test automation. They provide faster test execution and can be easily integrated into CI/CD pipelines.
Shift-Left Testing: The shift-left approach involves involving testers early in the development process. This helps in identifying issues and defects early on, reducing the overall time and effort required for testing.
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
As the field of test automation continues to evolve, Selenium remains at the forefront, providing a powerful and flexible framework for automating web browsers. By staying up-to-date with the latest developments and best practices, you can leverage Selenium to its full potential and achieve efficient and effective test automation.