As a software engineer, understanding RESTful APIs is essential for building modern and efficient applications. We will learn their purpose, structure, and how they can be leveraged to create powerful software solutions. We will provide you with a solid foundation to begin working with RESTful APIs.
What is a RESTful API?
A RESTful API, or Representational State Transfer, is an architectural style for designing networked applications. It provides a set of guidelines and constraints that enable systems to communicate over the internet. The main idea behind RESTful APIs is to use the existing protocols of the web, such as HTTP, to create a stateless communication between the client and the server.
RESTful APIs are based on the concept of resources, which are identified by unique URLs. These resources can be any kind of data, such as a user profile, a product listing, or a blog post. The client can interact with these resources by sending HTTP requests to the server, which then responds with the requested data or performs the requested action.
How do RESTful APIs work?
RESTful APIs work by using the HTTP protocol to perform CRUD operations (Create, Read, Update, Delete) on resources. The client sends an HTTP request to the server, specifying the desired operation and the URL of the resource. The server then processes the request and sends back an HTTP response, which contains the requested data or the result of the operation.
The Power Of Endpoints
To interact with a RESTful API, the client needs to know the available endpoints and the supported HTTP methods.
Endpoints are the URLs that represent the resources, and HTTP methods define the actions that can be performed on these resources.
The most common HTTP methods used in RESTful APIs are:
GET: Retrieves the representation of a resource
POST: Creates a new resource
PUT: Updates an existing resource
DELETE: Deletes a resource
The benefits of using RESTful APIs
There are several benefits to using RESTful APIs in software development. Firstly, RESTful APIs are easy to understand and use, as they follow a simple and consistent design pattern. This makes them highly scalable and allows developers to build complex systems with ease.
Another advantage of RESTful APIs is their statelessness. In a stateless architecture, each request from the client to the server is independent and does not rely on any previous requests. This makes the system more resilient and easier to maintain, as there is no need to store session data on the server.
RESTful APIs also promote loose coupling between the client and the server. The client does not need to know the internal details of the server, such as the database schema or the implementation of the server-side logic. This allows for better separation of concerns and enables different parts of the system to evolve independently.
Common HTTP methods used in RESTful APIs
To kick things off let’s see how a typical RESTful communication between a mobile app and a PaymentService API. would happen for a basic payment transaction.
For this usecase we can represent a sequence of operations like initiating a payment, checking the payment status, and receiving a confirmation of the payment.
This diagram illustrates the sequence of actions taken by a mobile app to interact with a payment service API, including initiating a payment, querying the payment status, and finally confirming the payment.
How Does It Work?
In RESTful APIs, HTTP methods are used to indicate the desired action to be performed on a resource. Each method has a specific purpose and should be used accordingly. Here are the most common HTTP methods used in RESTful APIs:
GET: This method is used to retrieve the representation of a resource. It should not have any side effects and should be idempotent, meaning that multiple identical requests should have the same effect as a single request.
POST: This method is used to create a new resource. It typically sends data in the request body and returns the newly created resource in the response.
PUT: This method is used to update an existing resource. It replaces the entire representation of the resource with the data sent in the request body.
DELETE: This method is used to delete a resource. It removes the resource from the server.
Key principles of RESTful API design
To design effective and efficient RESTful APIs, it is important to follow a set of key principles. These principles help ensure that the APIs are scalable, maintainable, and easy to use.
Here are some of the key principles of RESTful API design:
Resource-oriented architecture:
RESTful APIs are based on the concept of resources. Each resource should have a unique URL and should be accessed through standard HTTP methods.
Stateless communication:
RESTful APIs are stateless, meaning that each request from the client to the server should contain all the necessary information to perform the requested operation. The server does not store any session data.
Use of HTTP status codes:
RESTful APIs use HTTP status codes to indicate the result of a request. These status codes provide information about whether the request was successful, failed, or requires further action.
Consistent naming conventions:
RESTful APIs should use consistent and descriptive naming conventions for resources, endpoints, and URL parameters. This makes the APIs more intuitive and easier to understand.
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
Authentication and security in RESTful APIs
Authentication and security are critical aspects of RESTful API design. Without proper authentication and security measures, APIs can be vulnerable to unauthorized access and malicious attacks. Here are some best practices for implementing authentication and security in RESTful APIs:
Use HTTPS: Always use HTTPS (HTTP over SSL/TLS) to ensure secure communication between the client and the server. This encrypts the data transmitted over the network and prevents eavesdropping and tampering.
Authentication methods: Choose the appropriate authentication method based on your requirements. Common authentication methods used in RESTful APIs include API keys, OAuth, and JSON Web Tokens (JWT).
Authorization: Implement proper authorization mechanisms to control access to resources. Use roles and permissions to determine what actions a user can perform on a resource.
Rate limiting: Implement rate limiting to prevent abuse and protect your API from excessive requests. This ensures fair usage and helps maintain system performance.
Best practices for documenting RESTful APIs
Documenting RESTful APIs is crucial for their successful adoption and usage. Good documentation helps developers understand how to use the APIs effectively and reduces the learning curve.
Here are some best practices for documenting RESTful APIs:
Use a consistent format: Use a consistent format for documenting your APIs, such as OpenAPI (formerly known as Swagger) or API Blueprint. This makes it easier for developers to navigate and understand the documentation.
Provide clear and concise explanations: Clearly explain the purpose of each endpoint, the expected inputs and outputs, and any additional requirements or constraints.
Include code examples: Provide code examples in multiple programming languages to demonstrate how to use the API. This makes it easier for developers to integrate the API into their applications.
Keep the documentation up to date: Regularly update the documentation to reflect any changes or updates to the API. This ensures that developers always have access to the latest information.
Would you like to get engaged with professional Specialists?
We are a software development team with extensive development experience in the Hybrid and Crossplatform Applications development space. Let’s discuss your needs and requirements to find your best fit.
Tools and libraries for working with RESTful APIs
There are several tools and libraries available that can help you work with RESTful APIs more efficiently. These tools provide functionalities such as API testing, documentation generation, and client code generation. Here are some popular tools and libraries for working with RESTful APIs:
Postman: Postman is a powerful API testing tool that allows you to send requests to APIs and inspect the responses. It also provides features for automated testing and documentation generation.
Swagger: Swagger is a widely used framework for designing, building, and documenting RESTful APIs. It provides a set of tools for API development, including the ability to generate interactive documentation.
Retrofit: Retrofit is a popular HTTP client library for Android that simplifies the process of making HTTP requests to RESTful APIs. It provides a high-level API for managing network calls and parsing responses.
Axios: Axios is a JavaScript library that makes it easy to send HTTP requests from a browser or Node.js. It supports all major browsers and provides a simple and intuitive API.
Examples of popular RESTful APIs
RESTful APIs are widely used in various industries and domains. Here are some examples of popular RESTful APIs:
Twitter API: Twitter provides a RESTful API that allows developers to access and interact with Twitter’s data. The API provides endpoints for retrieving tweets, posting tweets, and performing other Twitter-related actions.
GitHub API: GitHub provides a RESTful API that allows developers to access and manipulate GitHub repositories, issues, and other resources. The API provides endpoints for managing repositories, creating pull requests, and performing other Git-related actions.
Stripe API: Stripe provides a RESTful API for processing online payments. The API allows developers to create and manage payments, subscriptions, and other payment-related operations.
Google Maps API: Google Maps provides a RESTful API that allows developers to embed maps, geocode addresses, and perform other location-related operations.
RESTFUL APIS USECASES
Data Retrieval
One of the most common use cases of RESTful APIs is retrieving data from a server. For example, let’s say you want to fetch a list of products from an e-commerce website. You can send a GET request to the API endpoint, and the server will respond with the requested data in JSON format.
GET /api/products
Data Creation
Another common use case is creating new data on the server. For instance, if you want to add a new user to a social media platform, you can send a POST request with the user’s information to the API endpoint.
POST /api/users
Data Update
Updating existing data is another typical use case. Let’s say you want to update a user’s profile picture. You can send a PUT request with the updated image file to the API endpoint.
PUT /api/users/{userId}
Data Deletion
Deleting data from the server is also a common use case. For example, if you want to delete a specific post from a blogging platform, you can send a DELETE request to the API endpoint.
DELETE /api/posts/{postId}
Now let’s see these Usecases in Action
To utilize a RESTful API, you use HTTP methods such as GET, POST, PUT, and DELETE to perform CRUD (Create, Read, Update, Delete) operations. Below is a brief overview of how each method is typically used, along with example code snippets in Python using the requests
library. Before you start, ensure you have the requests
library installed in your Python environment:
pip install requests
1. GET
The GET method is used to retrieve data from a specified resource.
Example:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
# Process the response
data = response.json()
print(data)
else:
print('Failed to retrieve data', response.status_code)
2. POST
The POST method is used to send data to a server to create a new resource.
Example:
import requests
url = 'https://api.example.com/data'
my_data = {'key': 'value'}
response = requests.post(url, json=my_data)
if response.status_code == 201:
print('Resource created successfully.')
else:
print('Failed to create resource', response.status_code)
3. PUT
The PUT method is used to update an existing resource or create a new one if it does not exist.
Example:
import requests
url = 'https://api.example.com/data/1' # Assuming '1' is the ID of the resource to update
updated_data = {'key': 'new value'}
response = requests.put(url, json=updated_data)
if response.status_code == 200:
print('Resource updated successfully.')
else:
print('Failed to update resource', response.status_code)
4. DELETE
The DELETE method is used to delete a specified resource.
Example:
import requests
url = 'https://api.example.com/data/1' # Assuming '1' is the ID of the resource to delete
response = requests.delete(url)
if response.status_code == 204:
print('Resource deleted successfully.')
else:
print('Failed to delete resource', response.status_code)
In these examples, replace 'https://api.example.com/data'
with the actual URL of the API you’re working with. Additionally, the specific status codes for success or failure might vary depending on the API’s design, so consult the API documentation for details on what to expect in response to your requests.
Setting Up Postman to Test Free Available APIs
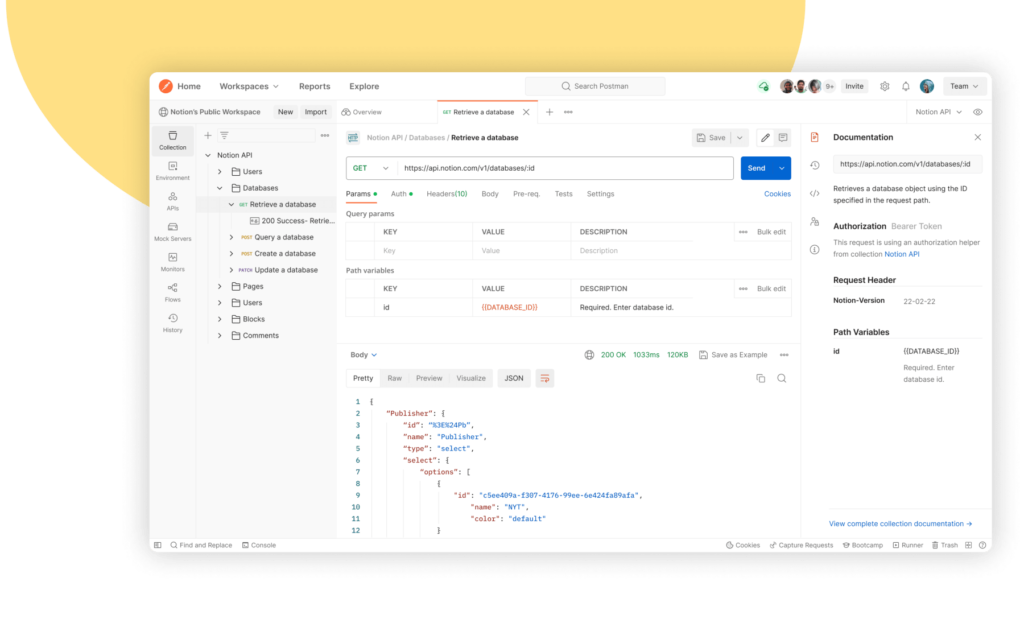
To test RESTful APIs, you can use tools like Postman. Here’s how you can set up Postman to test free available APIs:
- Download and install Postman from the official website.
- Launch Postman and create a new request.
- Enter the API endpoint URL in the request URL field.
- Select the appropriate HTTP method (GET, POST, PUT, DELETE) for your use case.
- Add any required headers or parameters.
- Click on the “Send” button to make the request and view the response.
By following these steps, you can easily test and interact with free available APIs using Postman.
RESTful APIs are versatile and widely used for various purposes, including data retrieval, creation, update, and deletion. With tools like Postman, you can easily test and experiment with different APIs to understand their functionality and integration possibilities.
RESTful APIs are essential tools for software engineers in building modern and efficient applications. They provide a standardized and scalable way to communicate between clients and servers.
By following the key principles of RESTful API design and implementing proper authentication and security measures, developers can create powerful software solutions that are robust and easy to use. So, whether you are a seasoned developer or just starting out, it’s time to unlock the power of RESTful APIs and take your engineering endeavors to the next level.
Now Go Start Using RESTful APIs
If you are just beginning your journey with RESTful APIs, RapidAPI offers an unmatched opportunity to experiment and learn. The platform facilitates easy testing of APIs, allowing developers to understand how different APIs behave and interact with their applications. This hands-on experience is crucial for building a solid foundation in API integration and development.
If you’re new to RapidAPI, this collection is a great place to start exploring APIs that are free to test,