Ruby is a dynamic, object-oriented programming language that has gained popularity among software engineers for its simplicity and versatility. Developed in the mid-1990s, Ruby has since become a go-to language for web development, data analysis, and automation tasks.
Why Choose Ruby?
One of the key reasons software engineers choose Ruby is its elegant syntax, which reads like plain English. This makes it easy to understand and write code, even for beginners. Additionally, Ruby’s flexibility allows developers to write concise and expressive code, leading to increased productivity and maintainability.
Use Cases for Ruby
Ruby can be used in a wide range of applications, making it a versatile language for software engineers. Here are some common use cases:
Web Development
Ruby on Rails, a popular web development framework built on Ruby, allows developers to quickly build robust and scalable web applications. Its convention-over-configuration approach minimizes the amount of code needed, resulting in faster development cycles.
Data Analysis
Ruby provides several libraries and frameworks, such as Nokogiri and Ruby Data, that make it easy to parse and analyze data. Whether you’re working with CSV files, XML documents, or web scraping, Ruby’s simplicity and rich ecosystem make it a great choice for data analysis tasks.
Automation
Ruby’s flexibility and ease of use make it ideal for automation tasks. Whether you need to automate repetitive tasks, build scripts, or create command-line tools, Ruby’s extensive standard library and community-driven gems provide the necessary tools to get the job done efficiently.

When Ruby is the right choice
The Ruby Programming Language: Best Practices and Design Patterns for Efficient Coding
Ruby has a number of popular frameworks that are widely used for web development. One of the most well-known frameworks is Ruby on Rails, also known as Rails. Rails is a full-stack web application framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of conventions and tools that make it easy to build web applications quickly.
Another popular framework is Sinatra, which is a lightweight web application framework. Sinatra is often used for smaller projects or APIs, as it provides a minimalistic approach to web development. It allows developers to quickly build web applications without the overhead of a full-stack framework like Rails.
Hanami is another framework that is gaining popularity in the Ruby community. Hanami follows the MVC architectural pattern and focuses on modularity and simplicity. It provides a set of tools and conventions that make it easy to build scalable and maintainable web applications.
In addition to frameworks, there are also a number of tools available for Ruby development. Popular text editors for Ruby include Sublime Text, Atom, and Visual Studio Code. These editors provide syntax highlighting, code completion, and other features that make it easier to write Ruby code.
For developers who prefer an integrated development environment (IDE), there are options like RubyMine and Aptana Studio. These IDEs provide a more comprehensive set of features, such as debugging, refactoring, and code analysis.
Version control is an important aspect of software development, and Git is the most widely used version control system. There are several Git clients available for Ruby developers, including command line tools like Git and GUI clients like SourceTree.
Ruby Syntax and Data Structures
- The syntax of Ruby is designed to be simple and easy to read. It is often described as having a natural language-like syntax, which makes it accessible to beginners and enjoyable for experienced developers. Here is a brief overview of the basic syntax of Ruby:
- Variables: In Ruby, variables are dynamically typed, which means that you don’t need to declare their type explicitly. You can assign a value to a variable by using the assignment operator (=).
- Strings: Strings in Ruby are enclosed in either single quotes (”) or double quotes (“”). They can contain any combination of characters, including special characters and escape sequences.
- Integers: Integers in Ruby are whole numbers without decimal points. They can be positive or negative.
- Arrays: Arrays in Ruby are ordered collections of objects. They can contain objects of any type, including other arrays.
- Hashes: Hashes in Ruby are collections of key-value pairs. They are similar to dictionaries in other programming languages.
- Ruby also provides a number of control structures that allow you to control the flow of your program. These include if/else statements, loops, and iterators. If/else statements allow you to execute different blocks of code based on a condition. Loops allow you to repeat a block of code a certain number of times or until a condition is met. Iterators allow you to iterate over collections and perform an action on each element.
Ruby Design Patterns: Overview and Implementation
Design patterns are reusable solutions to common problems that occur in software design. They provide a way to organize code and make it more maintainable and flexible. Ruby has a number of popular design patterns that are widely used in software development.
Some of the most commonly used design patterns in Ruby include the Singleton pattern, the Observer pattern, and the Decorator pattern.
The Singleton pattern is a creational design pattern that ensures that only one instance of a class is created. It is often used when you need to restrict the instantiation of a class to a single object. In Ruby, the Singleton pattern can be implemented by defining a class method that returns the same instance of the class every time it is called.
The Observer pattern is a behavioral design pattern that allows objects to be notified of changes in the state of other objects. It is often used when you need to maintain consistency between related objects. In Ruby, the Observer pattern can be implemented by defining an observer class that registers itself with the subject object and receives notifications when the subject’s state changes.
The Decorator pattern is a structural design pattern that allows behavior to be added to an object dynamically. It is often used when you need to add functionality to an object without modifying its structure. In Ruby, the Decorator pattern can be implemented by defining a decorator class that wraps another object and adds additional behavior to it.
Model-View-Controller (MVC) Design Pattern in Ruby
The Model-View-Controller (MVC) design pattern is widely used in web application development, and Ruby on Rails is built around this pattern. MVC separates the concerns of an application into three components: the model, the view, and the controller.
The model represents the data and business logic of the application. It is responsible for retrieving and manipulating data, as well as enforcing business rules. In Ruby on Rails, models are typically implemented as classes that inherit from ActiveRecord::Base, which provides a set of methods for interacting with a database.
The view is responsible for presenting data to the user. It is typically implemented as HTML templates that are rendered by the web server and sent to the client’s browser. In Ruby on Rails, views are implemented using ERB (Embedded Ruby), which allows you to embed Ruby code within HTML templates.
The controller is responsible for handling user requests and coordinating the interaction between the model and the view. It receives requests from the client’s browser, retrieves data from the model, and passes it to the view for rendering. In Ruby on Rails, controllers are implemented as classes that inherit from ActionController::Base, which provides a set of methods for handling HTTP requests.
The MVC design pattern provides a number of benefits for Ruby development. It promotes separation of concerns, which makes it easier to maintain and test code. It also allows for code reuse, as models and views can be shared across different parts of an application. Additionally, MVC provides a clear structure for organizing code, which makes it easier for developers to collaborate on a project.
Singleton Design Pattern in Ruby
The Singleton design pattern is a creational design pattern that ensures that only one instance of a class is created. It is often used when you need to restrict the instantiation of a class to a single object.
In Ruby, the Singleton pattern can be implemented by defining a class method that returns the same instance of the class every time it is called. Here is an example:
```ruby
class SingletonClass
def self.instance
@instance ||= new
end
private_class_method :new
end
singleton = SingletonClass.instance
```
In this example, the `instance` method is defined as a class method. It checks if the `@instance` variable is already set, and if not, it creates a new instance of the class. The `new` method is made private to prevent direct instantiation of the class.
The Singleton pattern can be useful in a variety of situations. For example, it can be used to manage a shared resource, such as a database connection or a logger. By ensuring that only one instance of the class is created, you can avoid conflicts and ensure that the resource is used consistently throughout your application.
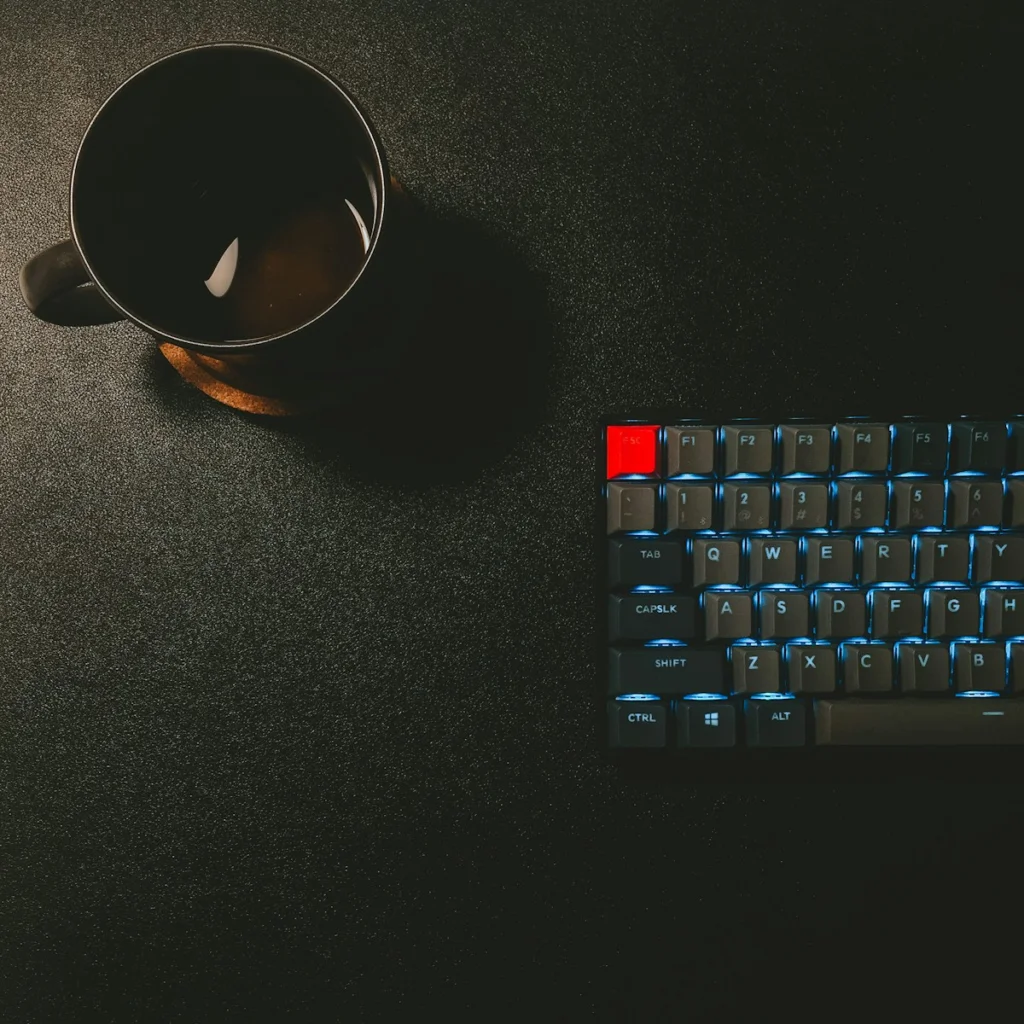
Ruby Patterns
Observer Design Pattern in Ruby
```ruby
class Subject
attr_reader :observers
def initialize
@observers = []
end
def add_observer(observer)
observers << observer
end
def remove_observer(observer)
observers.delete(observer)
end
def notify_observers
observers.each { |observer| observer.update }
end
end
class Observer
def update
puts "Subject's state has changed"
end
end
subject = Subject.new
observer = Observer.new
subject.add_observer(observer)
subject.notify_observers
```
In this example, the `Subject` class maintains a list of observers and provides methods for adding and removing observers. The `Observer` class defines an `update` method that is called when the subject’s state changes.
The Observer pattern can be useful in a variety of situations. For example, it can be used to implement event handling or to maintain consistency between different parts of an application. By decoupling the subject and the observers, you can make your code more flexible and maintainable.
Decorator Design Pattern in Ruby
The Decorator design pattern is a structural design pattern that allows behavior to be added to an object dynamically. It is often used when you need to add functionality to an object without modifying its structure.
In Ruby, the Decorator pattern can be implemented by defining a decorator class that wraps another object and adds additional behavior to it. Here is an example:
```ruby
class Component
def operation
"Component operation"
end
end
class Decorator
attr_reader :component
def initialize(component)
@component = component
end
def operation
"Decorator operation, #{component.operation}"
end
end
component = Component.new
decorator = Decorator.new(component)
puts decorator.operation
```
In this example, the `Component` class defines a basic operation. The `Decorator` class wraps a component and adds additional behavior to its operation.
The Decorator pattern can be useful in a variety of situations. For example, it can be used to add logging or caching functionality to an object without modifying its existing code. By using decorators, you can keep your code modular and reusable.
Ruby Best Practices: Code Organization and Documentation
Organizing your Ruby code in a clear and consistent manner is important for maintainability and readability. Here are some best practices for organizing your Ruby code:
– Use meaningful names for classes, modules, methods, and variables. This will make your code easier to understand and maintain.
– Follow the Single Responsibility Principle (SRP), which states that a class should have only one reason to change. Splitting your code into smaller, focused classes will make it easier to understand and test.
– Use modules to group related functionality. Modules can be included in classes to provide additional behavior.
– Use comments to explain the purpose and behavior of your code. Comments should be used sparingly and should focus on explaining why the code is written the way it is, rather than what it does.
– Use consistent indentation and formatting. This will make your code easier to read and understand.
Documentation is an important aspect of Ruby development. It helps other developers understand how to use your code and can serve as a reference for future maintenance. Here are some best practices for documenting your Ruby code:
– Use inline comments to explain complex or non-obvious parts of your code. Inline comments should be used sparingly and should focus on explaining why the code is written the way it is.
– Use documentation tools like YARD or RDoc to generate API documentation from your code. These tools allow you to write documentation in a structured format that can be easily parsed and displayed.
– Document public methods and classes using docstrings. Docstrings are special comments that describe the purpose, behavior, and usage of a method or class.
– Include examples and usage instructions in your documentation. This will help other developers understand how to use your code correctly.
Testing and Debugging in Ruby: Tools and Techniques
Testing is an important part of software development, as it helps ensure that your code works as expected and prevents regressions. In Ruby, there are several testing frameworks available, including RSpec and Minitest.
RSpec is a popular testing framework for Ruby that provides a domain-specific language (DSL) for writing tests. It allows you to write expressive, readable tests that describe the behavior of your code. RSpec provides a number of matchers and assertions that make it easy to write assertions about the state and behavior of your objects.
Minitest is another testing framework for Ruby that is included in the Ruby standard library. It provides a simple and lightweight testing framework that is easy to use. Minitest supports both unit tests and integration tests, and provides a number of assertions for testing different aspects of your code.
In addition to testing, debugging is an important skill for Ruby developers. Debugging allows you to identify and fix issues in your code. There are several tools available for debugging Ruby code, including byebug and pry.
Byebug is a command-line debugger for Ruby that allows you to set breakpoints, step through code, and inspect variables. It provides a simple and intuitive interface for debugging your code.
Pry is another popular debugging tool for Ruby that provides an interactive shell for exploring and debugging your code. Pry allows you to pause the execution of your program at any point and interactively explore the state of your objects.
In conclusion, Ruby is a powerful and flexible programming language that is popular among developers. It has a simple and elegant syntax, a vibrant community, and a number of frameworks and tools that make it easy to develop web applications.
Understanding Ruby design patterns and best practices is important for writing clean, maintainable code. Design patterns provide reusable solutions to common problems in software design, while best practices help organize your code and make it easier to understand and maintain.
By continuing to learn and explore Ruby development, you can become a more proficient developer and build high-quality applications. Whether you’re just starting out or have been programming in Ruby for years , there is always more to learn and discover. The Ruby community is vibrant and constantly evolving, with new libraries, frameworks, and best practices being developed all the time. By staying up-to-date with the latest trends and techniques, you can improve your coding skills and create more efficient and maintainable code. Additionally, by actively participating in the Ruby community, whether through attending meetups, joining online forums, or contributing to open-source projects, you can gain valuable insights from experienced developers and collaborate with others to solve complex problems. Ultimately, the more you invest in learning and exploring Ruby development, the more you will be able to leverage its power and create innovative and impactful applications.
FAQs
What is the Ruby programming language?
Ruby is a dynamic, object-oriented programming language that is designed to be easy to read and write. It was created in the mid-1990s by Yukihiro “Matz” Matsumoto and has since become popular for web development, scripting, and other applications.
What are some best practices for coding in Ruby?
Some best practices for coding in Ruby include using descriptive variable names, following the DRY (Don’t Repeat Yourself) principle, using single quotes for strings unless interpolation is needed, and using blocks and iterators instead of loops whenever possible.
What are design patterns in Ruby?
Design patterns in Ruby are reusable solutions to common programming problems. They are often used to improve code organization, maintainability, and scalability. Some common design patterns in Ruby include the Singleton pattern, the Observer pattern, and the Factory pattern.
Why is efficient coding important in Ruby?
Efficient coding in Ruby is important because it can improve the performance of your application, reduce memory usage, and make your code easier to maintain and debug. By following best practices and using design patterns, you can write code that is both efficient and effective.
What are some common mistakes to avoid when coding in Ruby?
Some common mistakes to avoid when coding in Ruby include using global variables, using unnecessary parentheses, using too many nested if statements, and not properly handling exceptions. It’s also important to avoid overcomplicating your code and to keep it as simple and readable as possible.