Java, the ubiquitous programming language, has been a mainstay in the developer world for nearly three decades. But where did it come from, and is it still relevant in 2024? Let’s dive into the world of Java, exploring its origins, current significance, and how you can join the Java journey.
From Sun Microsystems to Oracle: A Brief History
Java was born in 1995 under the visionary mind of James Gosling at Sun Microsystems. Designed to be “write once, run anywhere,” Java aimed to conquer platform-specific limitations. Oracle acquired Sun Microsystems in 2010, taking over the stewardship of Java.
Java’s Enduring Power in 2024
Despite the emergence of newer languages, Java remains a dominant force due to its:
- Platform independence: Java bytecode runs seamlessly across various platforms with the Java Virtual Machine (JVM).
- Large ecosystem: With extensive libraries, frameworks, and a vast community, finding solutions and support is effortless.
- Security: Java’s memory management and strong typing contribute to robust and secure applications.
- Object-oriented paradigm: Java promotes code reusability, maintainability, and modularity.
- Performance: With continuous optimizations, Java delivers reliable performance for demanding applications.
Who Should Learn Java?
If you’re considering a career in:
-
Enterprise software development: Java reigns supreme in this domain, powering mission-critical applications.
-
Android development: Android apps are primarily built with Java (or Kotlin, which compiles to Java bytecode).
-
Big data and analytics: Java plays a crucial role in processing and analyzing massive datasets.
-
Embedded systems: From smart TVs to medical devices, Java finds its way into various embedded systems.
-
General-purpose programming: Understanding Java opens doors to diverse programming opportunities.
Industries Embracing Java
Java’s versatility has attracted a wide range of industries, including:
- Finance: From banking applications to trading platforms, Java underpins financial operations.
- Healthcare: Electronic health records, medical imaging, and hospital management systems often rely on Java.
- Telecommunications: Network infrastructure, billing systems, and mobile applications frequently leverage Java.
- E-commerce: Leading e-commerce platforms utilize Java for their scalability and security.
- Education: Educational software, learning management systems, and online platforms commonly use Java.
Dedicated Full Stack Developers
Hiring Full Stack developers gives businesses access to pros proficient in various technologies and frameworks. Their versatility streamlines collaboration, leading to faster development and enhanced efficiency.
A Sample Java To-Do App with Explanations
This code demonstrates a basic To-Do application in Java, focusing on functionality rather than UI. It allows adding, marking tasks as done, and removing them.
1. Class definition:
public class TodoList {
private List<String> tasks;
public TodoList() {
this.tasks = new ArrayList<>();
}
// ... methods defined below
}
Here, we define a TodoList
class to manage our tasks. It holds a list of tasks represented as strings and has a constructor to initialize an empty list.
2. Adding a task:
public void addTask(String task) {
this.tasks.add(task);
System.out.println("Task added: " + task);
}
This method adds a new task (string) to the list and prints a confirmation message.
3. Marking a task as done:
public void markDone(String task) {
if (tasks.contains(task)) {
tasks.remove(task);
System.out.println("Task marked done: " + task);
} else {
System.out.println("Task not found: " + task);
}
}
This method checks if the given task exists in the list. If found, it removes it and prints a confirmation message. Otherwise, it indicates the task wasn’t found.
4. Removing a task:
public void removeTask(String task) {
if (tasks.contains(task)) {
tasks.remove(task);
System.out.println("Task removed: " + task);
} else {
System.out.println("Task not found: " + task);
}
}
Similar to marking done, this method removes the task if found and prints messages accordingly.
5. Listing all tasks:
public void listTasks() {
if (tasks.isEmpty()) {
System.out.println("No tasks in the list.");
} else {
System.out.println("Tasks:");
for (int i = 0; i < tasks.size(); i++) {
System.out.println((i + 1) + ". " + tasks.get(i));
}
}
}
This method iterates through the list and prints each task numbered for easy identification.
6. Usage example:
public static void main(String[] args) {
TodoList todoList = new TodoList();
todoList.addTask("Buy groceries");
todoList.addTask("Finish homework");
todoList.addTask("Call mom");
todoList.listTasks();
todoList.markDone("Buy groceries");
todoList.removeTask("Finish homework");
todoList.listTasks();
}
This code snippet demonstrates how to use the methods to add, mark done, remove, and list tasks.
Further Enhancements:
- You can modify the code to include features like:
- Marking tasks as priority
- Setting due dates
- Persisting tasks in a file or database
- Implementing a user interface
Would you like to get engaged with professional Specialists?
We are a software development team with extensive development experience in the Hybrid and Crossplatform Applications development space. Let’s discuss your needs and requirements to find your best fit.
Remember, this is a basic example to illustrate the logic. You can expand upon it to create a more robust and user-friendly application.
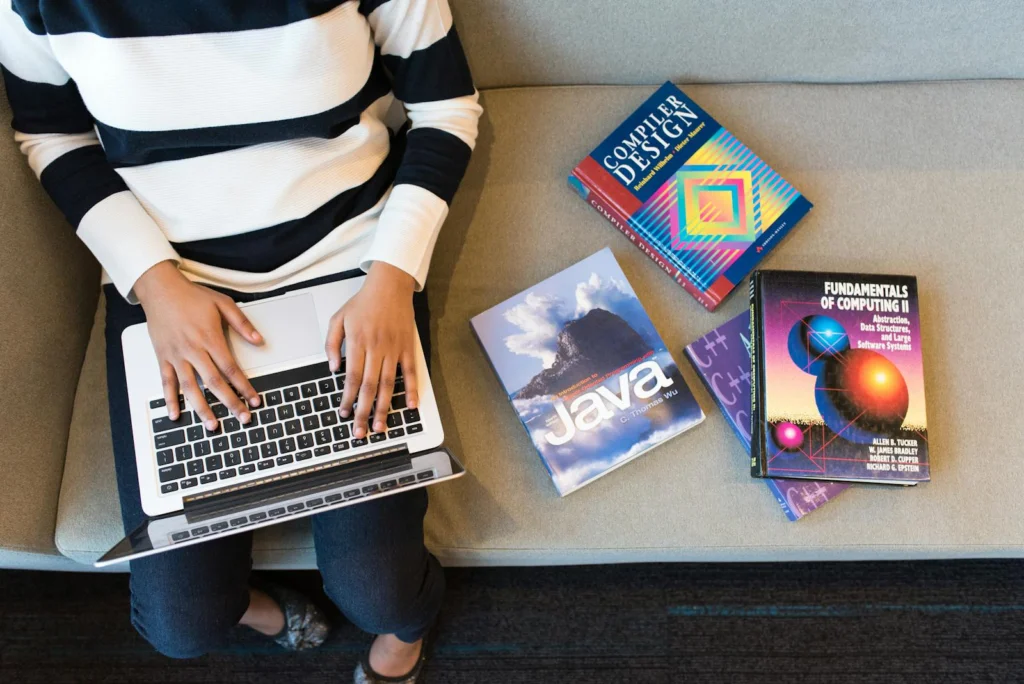
Where To Start Learning JAVA
Learning Java: Easier Than You Think
While Java has a reputation for being slightly more complex than beginner-friendly languages, its structured nature and abundant resources make it learnable. Here’s a roadmap to get you started:
Documentations:
- Official Java Tutorial: https://docs.oracle.com/cd/B13789_01/server.101/b10759/statements_5012.htm
- Java API Documentation: https://docs.oracle.com/en/java/javase/19
Videos:
- freeCodeCamp’s Java Tutorial for Beginners: https://www.youtube.com/watch?v=eIrMbAQSU34
- The Java MasterClass for Beginners 2023: https://www.udemy.com/topic/java/
Courses:
- University of Helsinki’s MOOC on Java Programming: https://java-programming.mooc.fi/
- Harvard’s CS50’s Introduction to Programming with Java: https://www.edx.org/learn/computer-science/harvard-university-cs50-s-introduction-to-computer-science
Remember:
- Start with the basics: Grasp core concepts like variables, operators, control flow, and object-oriented programming principles.
- Practice consistently: Coding regularly solidifies your understanding and builds confidence.
- Join the community: Online forums and communities offer invaluable support and guidance.
- Don’t hesitate to experiment: Explore different libraries, frameworks, and projects to broaden your skillset.
By following this roadmap and leveraging the plethora of free resources available, you’ll be well on your way to brewing your own cup of Java expertise!
Start your Java journey now!